..\CORE\core_cm3.h(90): error: #5: cannot open source input file "stdint.h": No such file or directory解决办法
时间: 2023-06-24 14:03:35 浏览: 184
这个错误提示是因为编译器无法找到 `stdint.h` 头文件。这个头文件是 C99 标准中定义的,包含了整数类型,如 `int32_t`,`uint16_t`等。
解决办法:
1. 确保你的编译器支持 C99 标准。如果不支持,可以在编译选项中添加 `-std=c99` 参数。
2. 如果编译器支持 C99 标准但仍然无法找到 `stdint.h` 头文件,可以尝试包含 `inttypes.h` 头文件,该头文件包含了 `stdint.h` 头文件。
3. 如果你使用的是 Keil MDK,那么可以尝试将 `stdint.h` 头文件添加到工程目录下,或者将其添加到 Keil 的文件夹路径中。
希望这些方法能帮助你解决问题。
相关问题
#include <iostream>#include <fstream>#include <string>int main() { std::string source_file_name, target_file_name; std::cout << "请输入源文件名:"; std::cin >> source_file_name; std::cout << "请输入目标文件名:"; std::cin >> target_file_name; std::ifstream source_file(source_file_name, std::ios::in | std::ios::binary); std::ofstream target_file(target_file_name, std::ios::out | std::ios::binary); if (!source_file || !target_file) { std::cerr << "文件打开失败" << std::endl; return 1; } target_file << source_file.rdbuf(); if (source_file.bad()) { std::cerr << "读取文件失败" << std::endl; source_file.close(); target_file.close(); return 1; } source_file.close(); target_file.close(); std::cout << "文件复制成功" << std::endl; return 0;}修改这段程序使他运行
这段程序本身是可以运行的,但是需要用户输入源文件名和目标文件名,也就是需要手动输入文件名才能进行复制操作。如果要实现自动化的文件复制,可以将源文件名和目标文件名写入程序中,例如:
```c++
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::string source_file_name = "source.txt"; // 源文件名
std::string target_file_name = "target.txt"; // 目标文件名
std::ifstream source_file(source_file_name, std::ios::in | std::ios::binary);
std::ofstream target_file(target_file_name, std::ios::out | std::ios::binary);
if (!source_file || !target_file) {
std::cerr << "文件打开失败" << std::endl;
return 1;
}
target_file << source_file.rdbuf();
if (source_file.bad()) {
std::cerr << "读取文件失败" << std::endl;
source_file.close();
target_file.close();
return 1;
}
source_file.close();
target_file.close();
std::cout << "文件复制成功" << std::endl;
return 0;
}
```
这样就可以直接运行程序进行文件复制操作,而不需要手动输入文件名。当然,如果需要复制其他文件,只需要修改源文件名和目标文件名即可。
修改这个程序的错误#include <iostream>#include <fstream>#include <string>int main() { std::string source_file_name, target_file_name; std::cout << "请输入源文件名:"; std::cin >> source_file_name; std::cout << "请输入目标文件名:"; std::cin >> target_file_name; std::ifstream source_file(source_file_name, std::ios::binary); std::ofstream target_file(target_file_name, std::ios::binary); if (!source_file || !target_file) { std::cerr << "文件打开失败" << std::endl; return 1; } target_file << source_file.rdbuf(); std::cout << "文件复制成功" << std::endl; return 0;}
这个程序主要是实现文件的复制功能,但是有一个小错误,应该将文件流对象source_file和target_file的打开方式改为 std::ios::in | std::ios::binary 和 std::ios::out | std::ios::binary,这样才能正确的读取和写入二进制文件。另外,建议在使用文件流对象时,对其进行异常判断和关闭操作,以保证程序的稳定性和安全性。修改后的程序如下所示:
```
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::string source_file_name, target_file_name;
std::cout << "请输入源文件名:";
std::cin >> source_file_name;
std::cout << "请输入目标文件名:";
std::cin >> target_file_name;
std::ifstream source_file(source_file_name, std::ios::in | std::ios::binary);
std::ofstream target_file(target_file_name, std::ios::out | std::ios::binary);
if (!source_file || !target_file) {
std::cerr << "文件打开失败" << std::endl;
return 1;
}
target_file << source_file.rdbuf();
if (source_file.bad()) {
std::cerr << "读取文件失败" << std::endl;
source_file.close();
target_file.close();
return 1;
}
source_file.close();
target_file.close();
std::cout << "文件复制成功" << std::endl;
return 0;
}
```
阅读全文
相关推荐
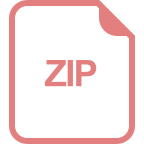
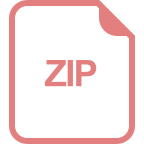
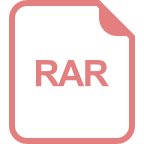














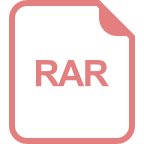