高斯模糊java代码_JAVA bitmap的高斯模糊代码
时间: 2023-12-09 12:03:51 浏览: 46
以下是Java中使用高斯模糊算法对Bitmap进行处理的代码示例:
```java
public static Bitmap gaussianBlur(Bitmap src, float radius) {
if (src == null) {
return null;
}
int width = src.getWidth();
int height = src.getHeight();
int[] pixels = new int[width * height];
src.getPixels(pixels, 0, width, 0, 0, width, height);
int[] resultPixels = new int[width * height];
System.arraycopy(pixels, 0, resultPixels, 0, pixels.length);
int[] alpha = new int[256];
for (int i = 0; i < 256; i++) {
alpha[i] = (int) (Math.exp(-((float) i * i) / (2 * radius * radius)) / (Math.sqrt(2 * Math.PI) * radius) * 255);
}
int[] box = new int[(int) (2 * radius)];
int boxWidth = (int) (radius / Math.sqrt(2 * Math.log(255)));
int boxSize = boxWidth * 2 + 1;
for (int i = 0; i < boxSize; i++) {
box[i] = alpha[(int) (255 * i / boxSize)];
}
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int red = 0;
int green = 0;
int blue = 0;
int alphaSum = 0;
for (int i = -boxWidth; i <= boxWidth; i++) {
int pixelIndex = x + clamp(i, 0, width - 1) + y * width;
int pixel = pixels[pixelIndex];
int coeff = box[Math.abs(i)];
red += Color.red(pixel) * coeff;
green += Color.green(pixel) * coeff;
blue += Color.blue(pixel) * coeff;
alphaSum += Color.alpha(pixel) * coeff;
}
int resultPixelIndex = x + y * width;
resultPixels[resultPixelIndex] = Color.argb(alphaSum >> 8, red >> 8, green >> 8, blue >> 8);
}
}
Bitmap result = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
result.setPixels(resultPixels, 0, width, 0, 0, width, height);
return result;
}
private static int clamp(int value, int min, int max) {
return Math.max(min, Math.min(value, max));
}
```
其中,使用了以下公式计算高斯函数的系数:
```
alpha[i] = exp(-i^2 / (2 * radius^2)) / (sqrt(2 * PI) * radius) * 255
```
对于每个像素点,都会计算出一个以该像素点为中心的正方形框,框内像素点的加权平均值作为该像素点的值。每个像素点的加权系数使用上述公式计算得到。在处理边缘像素点时,使用了clamp函数将超出边界的像素点坐标限制在合法范围内。最后,将处理后的像素数组生成为一个新的Bitmap并返回。
相关推荐
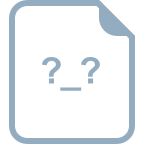
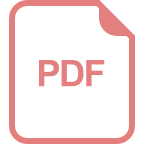
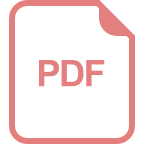











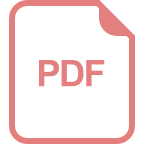
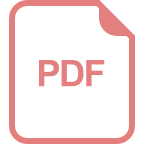
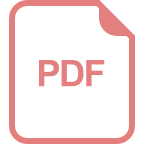