从txt文本中读取字符串并进行哈夫曼编码和解码重新读入到txt文本中
时间: 2024-05-07 16:21:48 浏览: 86
这里提供一个Python实现的示例代码。假设我们要对一个txt文本文件中的字符串进行哈夫曼编码和解码,并将结果重新写入txt文本文件中。
首先,我们需要实现哈夫曼编码和解码的函数。下面是一个简单的实现:
```python
import heapq
from collections import defaultdict
def huffman_encode(text):
freq = defaultdict(int)
for char in text:
freq[char] += 1
heap = [[weight, [char, ""]] for char, weight in freq.items()]
heapq.heapify(heap)
while len(heap) > 1:
low = heapq.heappop(heap)
high = heapq.heappop(heap)
for pair in low[1:]:
pair[1] = "0" + pair[1]
for pair in high[1:]:
pair[1] = "1" + pair[1]
heapq.heappush(heap, [low[0] + high[0]] + low[1:] + high[1:])
huffman_dict = dict(heapq.heappop(heap)[1:])
encoded_text = "".join([huffman_dict[char] for char in text])
return huffman_dict, encoded_text
def huffman_decode(encoded_text, huffman_dict):
inv_dict = {v: k for k, v in huffman_dict.items()}
decoded_text = ""
temp_code = ""
for bit in encoded_text:
temp_code += bit
if temp_code in inv_dict:
decoded_text += inv_dict[temp_code]
temp_code = ""
return decoded_text
```
接下来,我们可以读取txt文本文件中的字符串,进行编码和解码,并将结果重新写入txt文本文件中:
```python
# 读取文本文件中的字符串
with open("input.txt", "r") as f:
text = f.read()
# 进行哈夫曼编码
huffman_dict, encoded_text = huffman_encode(text)
# 将编码结果写入文本文件
with open("encoded.txt", "w") as f:
for char in encoded_text:
f.write(char)
# 读取编码结果
with open("encoded.txt", "r") as f:
encoded_text = f.read()
# 进行哈夫曼解码
decoded_text = huffman_decode(encoded_text, huffman_dict)
# 将解码结果写入文本文件
with open("decoded.txt", "w") as f:
f.write(decoded_text)
```
这样,我们就完成了对txt文本文件中字符串的哈夫曼编码和解码,同时将结果重新写入txt文本文件中。
阅读全文
相关推荐
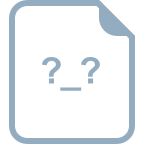

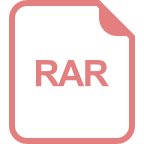
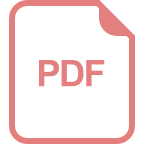
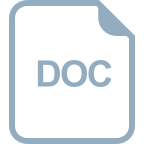
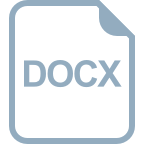
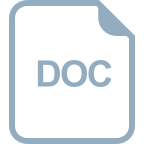
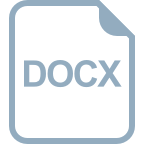
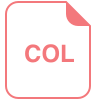




