如何用C++ 自己写出STOI函数
时间: 2024-02-03 19:14:03 浏览: 108
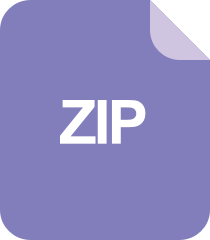
C++字符串函数_C++_
STOI函数是一个将字符串转换为整数的C++标准库函数。以下是一个简单的C++函数,可以自己实现STOI功能:
```c++
#include <iostream>
#include <string>
int stoi(const std::string& str) {
int result = 0;
bool isNegative = false;
// 处理负数情况
if (str[0] == '-') {
isNegative = true;
}
// 遍历字符串,计算数字结果
for (int i = isNegative ? 1 : 0; i < str.length(); i++) {
result = result * 10 + (str[i] - '0');
}
// 处理负数
if (isNegative) {
result *= -1;
}
return result;
}
int main() {
std::string str = "-123";
int num = stoi(str);
std::cout << num << std::endl;
return 0;
}
```
这个函数接受一个字符串参数,然后遍历字符串解析出数字结果。如果字符串以负号开头,将结果变为负数。最后将结果返回。
阅读全文
相关推荐
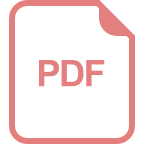
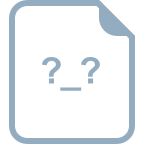

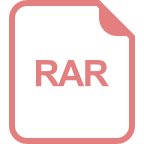














