帮我写一个使用栈和二叉树对中缀表达式求值的代码用C++写,借助二叉树和栈由中缀表达式创建二叉树,然后对二叉树进行后序遍历得到后缀表达式
时间: 2024-12-26 10:11:25 浏览: 3
在C++中,我们可以使用两个辅助数据结构:`std::stack`(栈)用于存储操作数,而`std::vector`或者自定义的二叉树节点类来表示二叉树。首先,我们需要定义一个简单的二叉树节点:
```cpp
#include <string>
#include <stack>
#include <vector>
class TreeNode {
public:
char op;
double num;
TreeNode *left, *right;
TreeNode(char op, double num) : op(op), num(num), left(NULL), right(NULL) {}
};
```
接下来,我们使用`infixToPostfix`函数将中缀表达式转换为后缀表达式:
```cpp
std::string infixToPostfix(const std::string &expr) {
std::string result = "";
std::stack<TreeNode*> st;
for (char c : expr) {
if (isdigit(c)) {
result += c; // 将数字直接添加到结果
} else if (c == '(' || c == ')' || c == '+' || c == '-') {
result += c; // 对于运算符,直接添加到结果
} else { // 遇到操作数,先将其压入栈,直到遇到其对应的右括号
while (!st.empty() && (!st.top()->op == '(' || st.top()->op > c))
result += " " + st.top()->num + " " + c, st.pop();
st.push(new TreeNode(c, 0)); // 保留操作符但暂存0作为数值
}
}
// 处理剩余的操作符
while (!st.empty()) {
result += " " + st.top()->num + " " + st.top()->op;
st.pop();
}
return result;
}
```
最后,我们将后缀表达式转换为计算结果:
```cpp
double evaluatePostfix(const std::string &postfix) {
std::stack<double> st;
for (char c : postfix) {
if (isdigit(c)) {
st.push(stoi(c));
} else { // 遇到操作符,弹出最近的两个操作数并计算
double b = st.top(); st.pop();
double a = st.top(); st.pop();
switch (c) {
case '+': st.push(a + b); break;
case '-': st.push(a - b); break;
case '*': st.push(a * b); break;
case '/': st.push(a / b); break;
}
}
}
return st.top(); // 返回最终结果
}
```
你可以通过下面的方式组合起来使用:
```cpp
int main() {
std::string infix = "3+5*2"; // 中缀表达式
std::string postfix = infixToPostfix(infix);
double result = evaluatePostfix(postfix);
std::cout << "Postfix expression: " << postfix << "\n";
std::cout << "Result: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
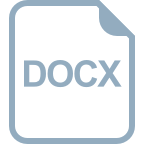
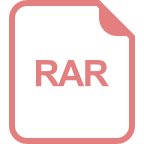
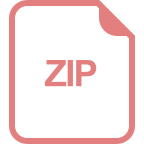
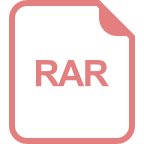
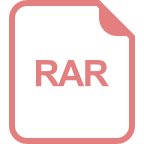











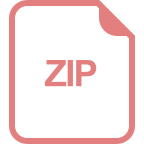
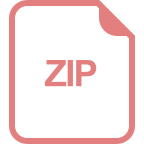