用c++实现程序实现aes加密和解密
时间: 2023-10-15 21:03:58 浏览: 76
好的,以下是一个使用Crypto++库实现AES加密和解密的示例代码:
```c++
#include <iostream>
#include <iomanip>
#include <string>
#include <cryptlib.h>
#include <aes.h>
#include <modes.h>
#include <base64.h>
using namespace CryptoPP;
std::string encryptAES(const std::string& plainText, const std::string& key)
{
std::string cipherText;
// 将密钥转换为byte数组
byte aesKey[AES::DEFAULT_KEYLENGTH];
::memcpy(aesKey, key.c_str(), key.size());
// 选择ECB加密模式
ECB_Mode<AES>::Encryption encryption(aesKey, sizeof(aesKey));
// 加密
StringSource(plainText, true,
new StreamTransformationFilter(encryption,
new StringSink(cipherText)
)
);
// 返回Base64编码后的密文
return base64_encode(reinterpret_cast<const unsigned char*>(cipherText.data()), cipherText.size());
}
std::string decryptAES(const std::string& cipherText, const std::string& key)
{
std::string plainText;
// 将密钥转换为byte数组
byte aesKey[AES::DEFAULT_KEYLENGTH];
::memcpy(aesKey, key.c_str(), key.size());
// 选择ECB加密模式
ECB_Mode<AES>::Decryption decryption(aesKey, sizeof(aesKey));
// 解密
StringSource(base64_decode(cipherText), true,
new StreamTransformationFilter(decryption,
new StringSink(plainText)
)
);
// 返回明文
return plainText;
}
int main()
{
// 待加密的明文和密钥
std::string plainText = "Hello, world!";
std::string key = "1234567890123456";
// 加密
std::string cipherText = encryptAES(plainText, key);
std::cout << "Cipher text: " << cipherText << std::endl;
// 解密
std::string decryptedText = decryptAES(cipherText, key);
std::cout << "Decrypted text: " << decryptedText << std::endl;
return 0;
}
```
这个示例代码使用了Crypto++库提供的AES加密和解密函数,使用ECB加密模式进行加密和解密,返回的是Base64编码后的密文。你可以根据自己的需求修改加密模式和返回结果的格式。
相关推荐
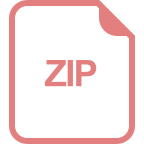
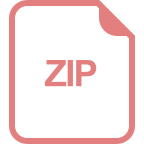
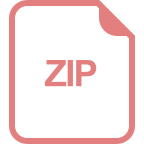














