用Python编写一个扫描文本的程序
时间: 2023-03-28 19:03:32 浏览: 60
你好,我是 CSDN 开发的 C 知道。关于你的问题,我可以回答。要用 Python 编写一个扫描文本的程序,你可以使用 Python 内置的文件操作函数,例如 open() 函数打开文件,read() 函数读取文件内容,以及正则表达式模块 re 来匹配文本中的特定模式。你可以先定义一个函数,接收文件路径作为参数,然后在函数内部打开文件,读取文件内容,使用正则表达式匹配需要的模式,最后返回匹配结果。希望这个回答能够帮到你。
相关问题
用Python写一个微信自动回复程序
当然可以!你可以使用Python编写一个微信自动回复程序。首先,你需要安装itchat库,它是一个专门用于微信的Python库。你可以使用以下命令在终端或命令提示符中安装itchat:
```
pip install itchat
```
然后,你可以使用以下代码编写一个简单的微信自动回复程序:
```python
import itchat
@itchat.msg_register(itchat.content.TEXT)
def reply(msg):
if msg['FromUserName'] == my_username:
return # 不回复自己的消息
itchat.send_msg('自动回复:我已经收到你的消息了,稍后会回复你!', toUserName=msg['FromUserName'])
if __name__ == '__main__':
itchat.auto_login(hotReload=True) # 登录微信
my_username = itchat.get_friends(update=True)[0]['UserName'] # 获取自己的用户名
itchat.run() # 运行微信
```
这段代码使用itchat库来实现微信自动回复功能。它会自动回复接收到的文本消息,并发送固定的回复内容"自动回复:我已经收到你的消息了,稍后会回复你!"。
请注意,在运行代码之前,你需要在手机上用微信扫描登录二维码。运行代码后,你的微信就会开始自动回复接收到的消息了。
希望这个代码能帮到你!如果有任何问题,请随时提问。
使用Python编写微信自动回复脚本
### 回答1:
为了编写微信自动回复脚本,我们可以使用itchat库,该库提供了对微信的接口,可以通过Python程序与微信进行交互。
以下是一个简单的微信自动回复脚本示例:
```python
import itchat
# 回复消息的函数
@itchat.msg_register(itchat.content.TEXT)
def reply_msg(msg):
if msg['FromUserName'] != myUserName:
itchat.send_msg('自动回复:' + msg['Text'], msg['FromUserName'])
# 登录微信
itchat.auto_login(hotReload=True)
# 获取自己的用户名
myUserName = itchat.get_friends(update=True)[0]["UserName"]
# 运行自动回复程序
itchat.run()
```
这个脚本中,我们使用`itchat.msg_register()`方法注册了一个消息处理函数`reply_msg()`,当收到文本消息时,该函数会自动回复消息内容,并附加上“自动回复”前缀。同时我们使用`itchat.auto_login()`方法登录微信,然后使用`itchat.get_friends()`方法获取自己的用户名,并保存到变量`myUserName`中。最后,我们使用`itchat.run()`方法启动自动回复程序。
值得注意的是,为了使该脚本能够长时间运行,我们需要在登录时设置`hotReload=True`,这样可以让登录状态得到保存,避免重复扫码登录的情况发生。
当我们运行该脚本后,微信中的好友发送消息时,程序会自动回复消息内容,并附加上“自动回复”前缀。
### 回答2:
使用Python编写微信自动回复脚本是可行的。首先,我们需要安装好Python的运行环境和相关的第三方库,如itchat。然后,通过编写Python脚本,我们可以实现对微信好友消息的监听和自动回复。
在脚本中,我们可以使用itchat库的API来实现微信的登录和消息的接收。通过捕获微信好友的文本消息,我们可以根据消息内容编写相应的逻辑来进行回复。例如,可以设置一些关键词回复,当好友发送包含特定关键词的消息时,自动回复相应的内容。
在编写脚本时,我们还可以使用正则表达式等高级技术来匹配和处理消息。例如,可以使用正则表达式来匹配特定格式的消息,并提取其中的信息用于回复。还可以利用其他Python的库来实现更复杂的逻辑,如将接收到的消息进行文本处理、分析等。
此外,我们还可以通过配置文件的方式,使脚本可以针对不同的好友或消息类型进行不同的回复。通过读取配置文件,可以灵活地设置回复规则,实现个性化的自动回复功能。
总结来说,使用Python编写微信自动回复脚本是一种方便且可行的方式。我们可以通过编写Python脚本,利用itchat库的API和其他Python的库,实现对微信好友消息的监听和自动回复,从而实现个性化和自动化的微信聊天机器人。
### 回答3:
使用Python编写微信自动回复脚本需要先安装一些必要的库,比如itchat,它可以模拟微信网页版的操作。
首先,在命令行中安装itchat库,可以使用pip命令:pip install itchat
然后,在Python脚本中导入itchat库:import itchat
接着,使用itchat库的login()函数登录微信账号:itchat.login()
登录成功后可以调用itchat库的函数获取微信好友列表:itchat.get_friends()
接下来,可以使用itchat库的run()函数开启微信消息监听模式,实现自动回复功能:itchat.run()
在run()函数中,可以使用装饰器@itchat.msg_register()来装饰自定义的函数,这个函数将会在收到好友消息时被调用。通过这个函数可以进行消息的处理和回复。
例如,我们可以定义一个回复消息的函数reply_to_friend(msg),其中msg是一个字典包含了收到的消息的具体信息。在函数的内部可以根据消息的类型进行逻辑判断和回复。
最后,运行整个Python脚本,扫描二维码登录微信账号后,即可实现自动回复功能。
需要注意的是,使用微信自动回复脚本有一定的限制,比如在非越狱的iPhone上,微信有防撤回机制,导致不能自动回复撤回消息。同时,使用自动回复脚本也需要谨慎,不要违反微信使用规定,以免被封号。
相关推荐
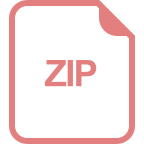
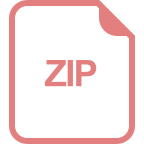

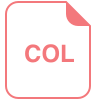
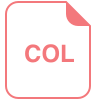

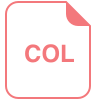







