无向图V(G1)={0,1,2,3,4,5,6,7,8,9,10} 边集合 E(G1)={(0,1),(0,2),(0,3),(1,4),(1,5),(2,5),(2,6),(3,7),(6,8),(6,9),(7,10)}关于这个无向图由用户输入一个结点的值,输出该结点的深度优先遍历和广度优先遍历序列的c语言代码
时间: 2023-06-19 09:05:26 浏览: 119
以下是深度优先遍历和广度优先遍历的C语言实现代码:
深度优先遍历:
```
#include <stdio.h>
#define MAX 11
int visited[MAX] = { 0 };
int graph[MAX][MAX] = {
{0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0},
{1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0},
{1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0},
{1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 0},
{0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0}
};
void DFS(int node) {
int i;
visited[node] = 1;
printf("%d ", node);
for (i = 0; i < MAX; i++) {
if (visited[i] == 0 && graph[node][i] == 1) {
DFS(i);
}
}
}
int main() {
int node;
printf("请输入要遍历的结点:");
scanf("%d", &node);
printf("深度优先遍历序列为:");
DFS(node);
return 0;
}
```
广度优先遍历:
```
#include <stdio.h>
#define MAX 11
int visited[MAX] = { 0 };
int graph[MAX][MAX] = {
{0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0},
{1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0},
{1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0},
{1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 0},
{0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0}
};
void BFS(int node) {
int queue[MAX], front = 0, rear = 0;
int i, j;
visited[node] = 1;
printf("%d ", node);
queue[rear++] = node;
while (front < rear) {
int temp = queue[front++];
for (i = 0; i < MAX; i++) {
if (visited[i] == 0 && graph[temp][i] == 1) {
visited[i] = 1;
printf("%d ", i);
queue[rear++] = i;
}
}
}
}
int main() {
int node;
printf("请输入要遍历的结点:");
scanf("%d", &node);
printf("广度优先遍历序列为:");
BFS(node);
return 0;
}
```
阅读全文
相关推荐
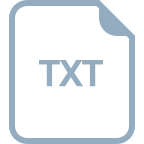
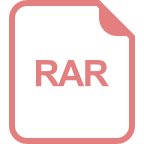

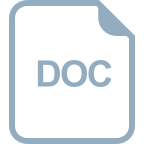







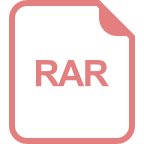
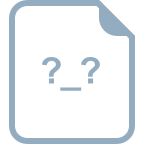