分析下面这个方法的功能def _make_divisible(v, divisor, min_value=None): """ This function is taken from the original tf repo. It ensures that all layers have a channel number that is divisible by 8 It can be seen here: https://github.com/tensorflow/models/blob/master/research/slim/nets/mobilenet/mobilenet.py """ if min_value is None: min_value = divisor new_v = max(min_value, int(v + divisor / 2) // divisor * divisor) # Make sure that round down does not go down by more than 10%. if new_v < 0.9 * v: new_v += divisor return new_v
时间: 2024-04-20 13:26:40 浏览: 33
这个方法的功能是确保所有的通道数量都可以被8整除。它接受三个参数:v代表原始通道数量,divisor代表除数,min_value代表最小值。如果没有提供最小值,则默认为除数。该方法会根据一定规则对原始通道数量进行调整,并返回一个新的通道数量。具体调整规则如下:
1. 将原始通道数量加上除数的一半,然后除以除数并取整数部分。
2. 将上一步得到的结果与最小值进行比较,取较大值。
3. 如果新的通道数量比原始通道数量的90%还要小,则将新的通道数量增加除数。
4. 返回最终调整后的通道数量。
这个方法的作用在于确保所有的层的通道数量都可以被8整除,这在一些模型设计中是很常见的要求。
相关问题
def _make_divisible(ch, divisor=8, min_ch=None):
if min_ch is None:
min_ch = divisor
# make sure that the number of channels is divisible by the divisor
new_ch = max(min_ch, int(ch + divisor / 2) // divisor * divisor)
# if the new number of channels is too small, increase it
if new_ch < 0.9 * ch:
new_ch += divisor
return new_ch
def _not_divisible(n): return lambda x: x % n > 0 这个函数中的x是哪来的?
这个函数 `_not_divisible(n)` 返回一个 lambda 函数,这个 lambda 函数接受一个参数 `x`,并且会判断 `x` 是否能被 `n` 整除。如果 `x` 不能被 `n` 整除,那么返回值是 True,否则返回值是 False。这个 lambda 函数中的 `x` 是在调用 `_not_divisible(n)` 返回的 lambda 函数时传入的参数。例如,如果我们定义了 `func = _not_divisible(3)`,那么调用 `func(4)` 时,`x` 的值是 4。
相关推荐
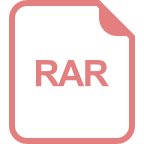
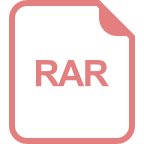













