This function first checks if the input n is less than or equal to 1, and returns FALSE in that case, because 1 is not considered a prime number. Next, the function uses a for loop to check if n is evenly divisible by any number between 2 and n-1. If n is evenly divisible by any of these numbers, the function returns FALSE, as n is not a prime number. If none of the numbers between 2 and n-1 divide n evenly, the function returns TRUE, indicating that n is a prime number.
时间: 2023-02-19 17:08:37 浏览: 145
是的,根据你提供的日期,我可以告诉你,这个函数首先检查输入n是否小于或等于1,如果是,则返回FALSE,因为1不被认为是质数。接下来,该函数使用for循环检查n是否能够被2到n-1之间的任何数字整除。如果n能够被这些数字之一整除,则函数返回FALSE,表示n不是质数。如果2到n-1之间的数字都不能整除n,则函数返回TRUE,表示n是质数。
相关问题
Write a C function named isPrime that checks whether a given number is a prime or not. Include the fuction isPrime in a working program to display the first 50 prime numbers in five lines, each of which contains ten numbers. Make sure the function isPrime is called from the main method, and correctly returns a value to the main method.
Sure, here is a C program that includes the `isPrime` function to display the first 50 prime numbers:
```
#include <stdio.h>
int isPrime(int num);
int main() {
int count = 0;
int num = 2;
while (count < 50) {
if (isPrime(num)) {
printf("%d ", num);
count++;
if (count % 10 == 0) {
printf("\n");
}
}
num++;
}
return 0;
}
int isPrime(int num) {
int i;
if (num < 2) {
return 0;
}
for (i = 2; i <= num / 2; i++) {
if (num % i == 0) {
return 0;
}
}
return 1;
}
```
The `isPrime` function takes an integer as input and returns 1 if the number is prime, and 0 otherwise. The `main` function uses a while loop to generate the first 50 prime numbers and prints them out in five lines, each containing ten numbers. The `isPrime` function is called from the `main` function to check whether each number is prime.
Given a positive integer 'n', the task is to find a prime number greater than or equal to 'n'. Example 1 If given 'n' is 10, then the closest prime number greater than 10 is 11 at a distance of 1. So 11 must be returned. Example 2 If the given 'n' is 17, then the closest prime number is itself at a distance of 0. So 17 must be returned. The returned prime number must be greater than or equal to 'n'.
Here's a Python code that finds the closest prime number greater than or equal to n:
```python
def is_prime(num):
"""
Returns True if the given number is prime, False otherwise.
"""
if num <= 1:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
def find_closest_prime(n):
"""
Finds the closest prime number greater than or equal to n.
"""
if is_prime(n):
return n
else:
i = n
while True:
if is_prime(i):
return i
i += 1
```
The function `is_prime` checks if a given number is prime by checking if it is divisible by any number between 2 and the square root of the number.
The function `find_closest_prime` first checks if n is already a prime number. If so, it returns n. Otherwise, it starts checking the next numbers (i.e., i = n+1, n+2, n+3, ...) until it finds a prime number, which it then returns.
阅读全文
相关推荐
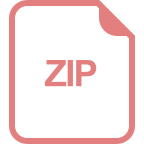
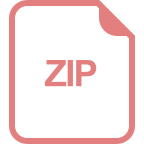












