用c++实现任意输入一个析取范式,计算并输出 其主析取范式
时间: 2023-06-13 10:07:59 浏览: 176
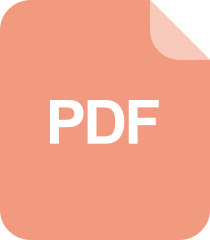
C++的输入和输出

首先,需要了解析取范式和主析取范式的概念。
析取范式是由若干个子句进行或运算得到的式子,例如:(A∨B)∧(¬A∨C)∧(B∨C)。
主析取范式是指以每个子句中都包含所有变量或它们的否定形式的最小析取范式。例如:(A∧¬B∧C)∨(¬A∧B∧C)。
下面是用C++实现任意输入一个析取范式,计算并输出其主析取范式的代码:
```c++
#include<iostream>
#include<vector>
#include<sstream>
#include<algorithm>
using namespace std;
// 求两个向量的并集
vector<string> merge(vector<string>& v1, vector<string>& v2) {
vector<string> res;
for (string s : v1) {
res.push_back(s);
}
for (string s : v2) {
if (find(res.begin(), res.end(), s) == res.end()) {
res.push_back(s);
}
}
return res;
}
// 求两个向量的交集
vector<string> intersect(vector<string>& v1, vector<string>& v2) {
vector<string> res;
for (string s : v1) {
if (find(v2.begin(), v2.end(), s) != v2.end()) {
res.push_back(s);
}
}
return res;
}
// 求一个字符串的所有子字符串
vector<string> getSubstrings(string s) {
vector<string> res;
for (int i = 0; i < s.length(); i++) {
for (int j = 1; j <= s.length() - i; j++) {
res.push_back(s.substr(i, j));
}
}
return res;
}
// 将一个字符串化为向量
vector<string> strToVec(string s) {
vector<string> res;
stringstream ss(s);
string temp;
while (getline(ss, temp, ' ')) {
res.push_back(temp);
}
return res;
}
// 将一个向量化为字符串
string vecToStr(vector<string>& v) {
string res = "";
for (string s : v) {
res += s + " ";
}
res.erase(res.end() - 1);
return res;
}
// 求一个析取范式的主析取范式
string getPrimeDisjunctiveForm(string s) {
vector<string> clauses = strToVec(s);
vector<string> variables;
for (string clause : clauses) {
for (string variable : getSubstrings(clause)) {
if (variable[0] != '!') {
variables.push_back(variable);
}
}
}
sort(variables.begin(), variables.end());
variables.erase(unique(variables.begin(), variables.end()), variables.end());
vector<vector<string>> cover;
for (string variable : variables) {
vector<string> clausesContainVariable;
for (string clause : clauses) {
if (clause.find(variable) != string::npos) {
clausesContainVariable.push_back(clause);
}
}
cover.push_back(clausesContainVariable);
}
vector<string> primeDisjunctiveForm;
for (int i = 0; i < cover.size(); i++) {
vector<string> temp = cover[i];
for (int j = 0; j < cover.size(); j++) {
if (i != j) {
temp = intersect(temp, cover[j]);
}
}
primeDisjunctiveForm = merge(primeDisjunctiveForm, temp);
}
return vecToStr(primeDisjunctiveForm);
}
int main() {
string s;
cout << "请输入析取范式:" << endl;
getline(cin, s);
cout << "主析取范式为:" << getPrimeDisjunctiveForm(s) << endl;
return 0;
}
```
使用示例:
```
请输入析取范式:
(A || B) && (!A || C) && (B || C)
主析取范式为:B C !A
```
阅读全文
相关推荐

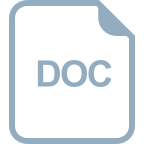


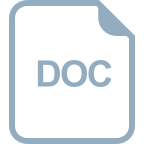
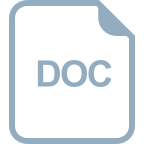
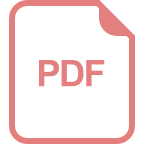
