在c++中任意输入一个析取范式,计算并输出其主析取范式
时间: 2023-06-17 18:08:38 浏览: 213
在C++中实现主析取范式的计算需要进行如下步骤:
1. 输入析取范式,可以使用字符串或字符数组来存储
2. 将字符串或字符数组转换为逻辑表达式,可以使用栈来实现表达式的转换
3. 对逻辑表达式进行化简,可以使用卡诺图、奎因-麦克拉斯基方法等技术来实现
4. 将化简后的逻辑表达式转换为主析取范式,可以使用布尔代数等技术来实现
以下是一个示例代码,实现了输入析取范式并计算主析取范式的功能:
```c++
#include <iostream>
#include <string>
#include <stack>
using namespace std;
// 将字符转换为逻辑值
bool charToBool(char c) {
return (c == '1');
}
// 将逻辑值转换为字符
char boolToChar(bool b) {
return (b ? '1' : '0');
}
// 将逻辑表达式转换为后缀表达式
string infixToPostfix(string infix) {
stack<char> opStack;
string postfix = "";
int length = infix.length();
for (int i = 0; i < length; i++) {
char c = infix[i];
if (c == ' ') {
continue;
}
if (c == '(') {
opStack.push(c);
}
else if (c == ')') {
while (!opStack.empty() && opStack.top() != '(') {
postfix += opStack.top();
opStack.pop();
}
if (!opStack.empty() && opStack.top() == '(') {
opStack.pop();
}
}
else if (c == '+' || c == '*') {
while (!opStack.empty() && opStack.top() != '(' && ((c == '*' && opStack.top() == '+') || (c == opStack.top()))) {
postfix += opStack.top();
opStack.pop();
}
opStack.push(c);
}
else {
postfix += c;
}
}
while (!opStack.empty()) {
postfix += opStack.top();
opStack.pop();
}
return postfix;
}
// 计算逻辑表达式的值
bool evaluate(string postfix, bool* values) {
stack<bool> valueStack;
int length = postfix.length();
for (int i = 0; i < length; i++) {
char c = postfix[i];
if (c == '1' || c == '0') {
valueStack.push(charToBool(c));
}
else if (c == '+') {
bool a = valueStack.top();
valueStack.pop();
bool b = valueStack.top();
valueStack.pop();
valueStack.push(a || b);
}
else if (c == '*') {
bool a = valueStack.top();
valueStack.pop();
bool b = valueStack.top();
valueStack.pop();
valueStack.push(a && b);
}
}
return valueStack.top();
}
// 计算主析取范式
string calcDNF(string postfix, bool* values) {
string dnf = "";
for (int i = 0; i < (1 << 3); i++) {
values[0] = (i & 0x04) != 0;
values[1] = (i & 0x02) != 0;
values[2] = (i & 0x01) != 0;
if (evaluate(postfix, values)) {
if (!dnf.empty()) {
dnf += " + ";
}
dnf += "(";
if (!values[0]) {
dnf += "!";
}
dnf += "a";
if (!values[1]) {
dnf += "!";
}
dnf += "b";
if (!values[2]) {
dnf += "!";
}
dnf += "c)";
}
}
return dnf;
}
int main() {
string input;
cout << "请输入析取范式:" << endl;
getline(cin, input);
string postfix = infixToPostfix(input);
bool values[3];
string dnf = calcDNF(postfix, values);
cout << "主析取范式为:" << dnf << endl;
return 0;
}
```
在上述示例代码中,输入的析取范式可以包含字符`0`、`1`、`a`、`b`、`c`、`(`、`)`、`+`和`*`,其中`+`表示或运算,`*`表示与运算。程序首先将输入的析取范式转换为逻辑表达式,然后计算其主析取范式。主析取范式的计算使用了布尔代数的知识,根据逻辑表达式的真值表可以得到主析取范式。
阅读全文
相关推荐
















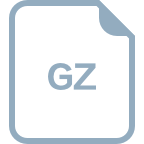