我需要一段哈希表的c++代码
时间: 2023-10-17 11:11:53 浏览: 139
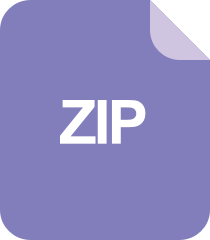
C++期末设计作业,哈希表的构建(源代码+文档说明)
以下是一个基于链表的哈希表的C代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define HASH_SIZE 1000
typedef struct Node {
char *key;
int value;
struct Node *next;
} Node;
Node *hashTable[HASH_SIZE];
// 哈希函数
unsigned int hash(const char *key) {
unsigned long hashVal = 0;
while(*key != '\0') {
hashVal = (hashVal << 4) + *key++;
unsigned long g = hashVal & 0xF0000000;
if (g != 0) {
hashVal ^= g >> 24;
}
hashVal &= ~g;
}
return hashVal % HASH_SIZE;
}
// 查找键对应的值
int get(const char *key) {
unsigned int pos = hash(key);
Node *p = hashTable[pos];
while(p != NULL) {
if(strcmp(p->key, key) == 0) {
return p->value;
} else {
p = p->next;
}
}
return -1;
}
// 插入键值对
void put(const char *key, int value) {
unsigned int pos = hash(key);
Node *p = hashTable[pos];
while(p != NULL) {
if(strcmp(p->key, key) == 0) {
p->value = value;
return;
} else {
p = p->next;
}
}
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->key = (char*)malloc(strlen(key) + 1);
strcpy(newNode->key, key);
newNode->value = value;
newNode->next = hashTable[pos];
hashTable[pos] = newNode;
}
int main() {
put("apple", 5);
put("orange", 8);
printf("%d\n", get("apple"));
printf("%d\n", get("banana"));
return 0;
}
```
阅读全文
相关推荐
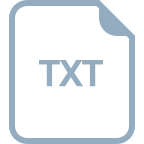
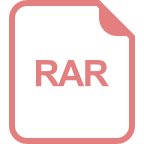

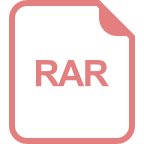
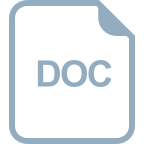
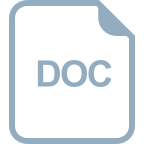
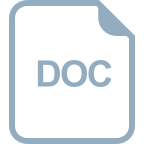
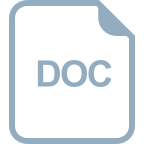
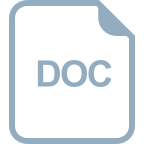
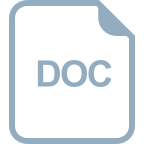
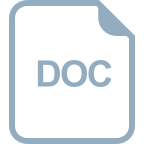
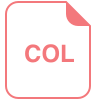
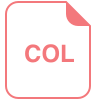
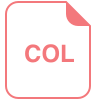
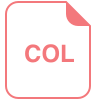

