'生成Delaunay三角网 Dim mesh As TriangleNet.Mesh = Nothing If input IsNot Nothing Then mesh = CType(TriangleNet.Geometry.ExtensionMethods.Triangulate(input, options), TriangleNet.Mesh) End If 怎么计算任意平面坐标点P(X,Y)的高程
时间: 2024-02-14 17:32:39 浏览: 26
计算任意平面坐标点 P(X,Y) 的高程通常需要使用插值算法。一种常用的插值算法是基于三角网格的插值算法,如线性插值或二次插值等。
在使用三角网格插值算法进行高程计算时,需要首先将输入的坐标点 P(X,Y) 插入到三角网格中。这可以通过将 P(X,Y) 添加到网格的顶点列表中,然后重新计算三角剖分来实现。
一旦 P(X,Y) 被插入到三角网格中,就可以使用插值算法计算其高程。例如,使用线性插值算法,可以计算 P(X,Y) 在其所在的三角形内的高程值。具体方法是,首先确定 P(X,Y) 所在的三角形,然后在该三角形的三个顶点处获取高程值,并使用这些值进行线性插值计算。
如果需要更精确的高程计算,可以使用二次插值或更高阶的插值方法。这些方法通常需要在三角形中使用更多的顶点来提高插值的准确性。
相关问题
Coding me scipy.spatial.Delaunay create mesh
Sure, here is an example code for creating a mesh using `scipy.spatial.Delaunay`:
```python
import numpy as np
from scipy.spatial import Delaunay
# Define the points for triangulation
points = np.array([[0, 0], [0, 1], [1, 0], [1, 1], [0.5, 0.5]])
# Compute the Delaunay triangulation
tri = Delaunay(points)
# Extract the triangles and vertices of the mesh
triangles = points[tri.simplices]
vertices = triangles.reshape(-1, 2)
# Print the vertices of the mesh
print(vertices)
```
In this example, we first define some points in a 2D space. We then use `scipy.spatial.Delaunay` to compute the Delaunay triangulation of these points, which creates a mesh of non-overlapping triangles covering the space. Finally, we extract the vertices of the triangles to obtain the full set of vertices for the mesh. You can modify the `points` array to create different meshes for your specific application.
用scipy.spatial.Delaunay绘制曲面三角网格的三维立体图
首先,我们需要生成一些三维坐标点作为曲面的数据。我们可以使用NumPy库中的meshgrid函数来生成网格坐标点,然后将其转换为一维数组。
```python
import numpy as np
from scipy.spatial import Delaunay
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
# 生成网格坐标点
x, y = np.meshgrid(np.linspace(-1, 1, 10), np.linspace(-1, 1, 10))
z = x**2 + y**2
# 将坐标点转换为一维数组
points = np.vstack([x.ravel(), y.ravel(), z.ravel()]).T
```
接下来,我们使用Delaunay函数来生成三角剖分,这将返回一个包含所有三角形顶点索引的数组。
```python
tri = Delaunay(points)
```
然后,我们可以使用Poly3DCollection函数来绘制三角形。这个函数需要一个由三角形顶点组成的二维数组,我们可以从Delaunay函数返回的数组中提取这些顶点。
```python
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制三角网格
verts = [points[tri.simplices[i]] for i in range(len(tri.simplices))]
ax.add_collection(Poly3DCollection(verts, facecolors='w', edgecolors='k', alpha=0.25))
# 添加坐标轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
完整代码如下:
```python
import numpy as np
from scipy.spatial import Delaunay
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
# 生成网格坐标点
x, y = np.meshgrid(np.linspace(-1, 1, 10), np.linspace(-1, 1, 10))
z = x**2 + y**2
# 将坐标点转换为一维数组
points = np.vstack([x.ravel(), y.ravel(), z.ravel()]).T
# 生成三角剖分
tri = Delaunay(points)
# 绘制三角网格
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
verts = [points[tri.simplices[i]] for i in range(len(tri.simplices))]
ax.add_collection(Poly3DCollection(verts, facecolors='w', edgecolors='k', alpha=0.25))
# 添加坐标轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
plt.show()
```
相关推荐
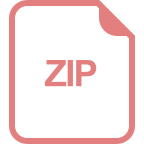












