怎么控制虚拟轴值的增长速度
时间: 2024-04-22 08:23:35 浏览: 36
要控制虚拟轴值的增长速度,可以通过以下几种方法实现:
1. 在Unity的Input Manager中创建一个新的虚拟轴,并将其绑定到水平移动的按键或手柄摇杆。然后,在角色控制器的脚本中使用`Input.GetAxisRaw()`方法获取虚拟轴的原始值,而不是`Input.GetAxis()`方法获取虚拟轴的平滑值。这样可以避免虚拟轴值平滑增长的效果,而是直接根据按键或手柄摇杆的输入值来改变虚拟轴的值。
```csharp
public float moveSpeed = 5f; // 移动速度
public float moveAcceleration = 10f; // 移动加速度
public float moveDeceleration = 10f; // 移动减速度
private float moveInput;
private Rigidbody2D rb;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
// 获取虚拟轴的原始值
moveInput = Input.GetAxisRaw("Horizontal");
// 根据虚拟轴值进行移动
if (moveInput != 0)
{
// 根据虚拟轴值和移动加速度计算速度增量
float velocityIncrement = moveInput * moveAcceleration * Time.fixedDeltaTime;
// 将速度增量限制在移动速度范围内
float newVelocityX = Mathf.Clamp(rb.velocity.x + velocityIncrement, -moveSpeed, moveSpeed);
// 将刚体的速度设置为新的速度
rb.velocity = new Vector2(newVelocityX, rb.velocity.y);
}
else
{
// 根据移动减速度逐渐减慢速度
float velocityDecrement = moveDeceleration * Time.fixedDeltaTime;
float newVelocityX = Mathf.MoveTowards(rb.velocity.x, 0f, velocityDecrement);
rb.velocity = new Vector2(newVelocityX, rb.velocity.y);
}
}
```
以上代码会根据虚拟轴的原始值来计算速度增量,然后将速度增量限制在移动速度范围内,并将刚体的速度设置为新的速度。如果虚拟轴的原始值为零,则根据移动减速度逐渐减慢速度,从而实现缓慢停止的效果。
2. 在角色控制器的脚本中添加一个移动速度的插值变量,用于控制虚拟轴值的增长速度。当按下移动键时,将插值变量从零逐渐增加到移动速度,从而实现缓慢加速的效果。当松开移动键时,将插值变量从当前速度逐渐减少到零,从而实现缓慢停止的效果。
```csharp
public float moveSpeed = 5f; // 移动速度
public float moveAcceleration = 10f; // 移动加速度
public float moveDeceleration = 10f; // 移动减速度
private float moveInput;
private float moveSpeedLerp = 0f;
private Rigidbody2D rb;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
// 获取虚拟轴的原始值
moveInput = Input.GetAxisRaw("Horizontal");
// 根据虚拟轴值进行移动
if (moveInput != 0)
{
// 根据插值变量和移动速度计算速度增量
float velocityIncrement = moveSpeedLerp * moveSpeed * Time.fixedDeltaTime;
// 将速度增量限制在移动速度范围内
float newVelocityX = Mathf.Clamp(rb.velocity.x + velocityIncrement, -moveSpeed, moveSpeed);
// 将刚体的速度设置为新的速度
rb.velocity = new Vector2(newVelocityX, rb.velocity.y);
// 逐渐增加插值变量
moveSpeedLerp = Mathf.MoveTowards(moveSpeedLerp, 1f, moveAcceleration * Time.fixedDeltaTime);
}
else
{
// 根据插值变量和移动速度计算速度减量
float velocityDecrement = moveSpeedLerp * moveSpeed * moveDeceleration * Time.fixedDeltaTime;
// 将速度减量限制在移动速度范围内
float newVelocityX = Mathf.Clamp(rb.velocity.x - velocityDecrement, -moveSpeed, moveSpeed);
// 将刚体的速度设置为新的速度
rb.velocity = new Vector2(newVelocityX, rb.velocity.y);
// 逐渐减少插值变量
moveSpeedLerp = Mathf.MoveTowards(moveSpeedLerp, 0f, moveDeceleration * Time.fixedDeltaTime);
}
}
```
以上代码会根据插值变量和移动速度计算速度增量或减量,并将速度增量或减量限制在移动速度范围内,并将刚体的速度设置为新的速度。如果虚拟轴的原始值为零,则根据移动减速度逐渐减少插值变量,从而实现缓慢停止的效果。
相关推荐
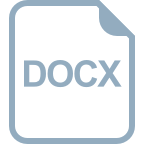
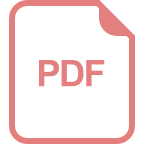
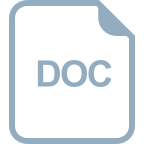
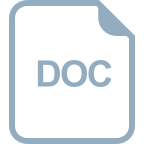
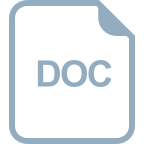
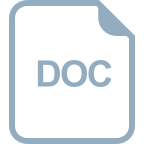
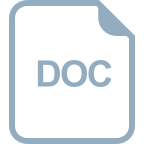
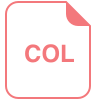








