数据结构实现含括号1位数的算术四则运算的表达式求值
时间: 2023-11-19 09:06:55 浏览: 83
首先需要将算术表达式转换为后缀表达式,然后再利用栈来求值。
算法步骤如下:
1. 定义一个运算符优先级的字典,方便后面判断。
2. 定义一个栈,用于存储操作数和运算符。
3. 遍历算术表达式中的每一个字符,如果是数字,则直接压入栈中;如果是运算符,则进行以下操作:
- 如果栈为空或者栈顶元素为左括号,直接将运算符压入栈中。
- 如果运算符优先级大于栈顶运算符优先级,则将运算符压入栈中。
- 如果运算符优先级小于等于栈顶运算符优先级,则将栈顶运算符弹出并计算,直到栈顶运算符优先级小于当前运算符优先级,然后再将当前运算符压入栈中。
4. 如果遍历完整个算术表达式后,栈不为空,则继续弹出栈顶元素并计算,直到栈为空。
5. 返回最终的计算结果。
代码实现如下:
```python
def evaluate(expression):
precedence = {'+': 1, '-': 1, '*': 2, '/': 2}
stack = []
for char in expression:
if char.isdigit():
stack.append(int(char))
elif char in precedence:
while stack and stack[-1] != '(' and precedence[char] <= precedence.get(stack[-1], 0):
operator = stack.pop()
operand2 = stack.pop()
operand1 = stack.pop()
if operator == '+':
stack.append(operand1 + operand2)
elif operator == '-':
stack.append(operand1 - operand2)
elif operator == '*':
stack.append(operand1 * operand2)
elif operator == '/':
stack.append(operand1 / operand2)
stack.append(char)
elif char == '(':
stack.append(char)
elif char == ')':
while stack and stack[-1] != '(':
operator = stack.pop()
operand2 = stack.pop()
operand1 = stack.pop()
if operator == '+':
stack.append(operand1 + operand2)
elif operator == '-':
stack.append(operand1 - operand2)
elif operator == '*':
stack.append(operand1 * operand2)
elif operator == '/':
stack.append(operand1 / operand2)
stack.pop()
while stack:
operator = stack.pop()
operand2 = stack.pop()
operand1 = stack.pop()
if operator == '+':
stack.append(operand1 + operand2)
elif operator == '-':
stack.append(operand1 - operand2)
elif operator == '*':
stack.append(operand1 * operand2)
elif operator == '/':
stack.append(operand1 / operand2)
return stack[0]
```
测试代码如下:
```python
expression = '(1+2)*3-4/2'
result = evaluate(expression)
print(result) # 输出 7.0
```
注意:以上代码只适用于含括号1位数的算术四则运算,对于多位数或者其他特殊情况需要进行相应的修改。
阅读全文
相关推荐
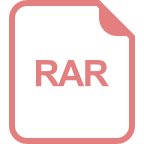

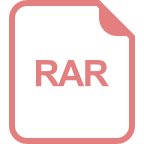
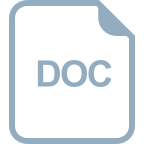
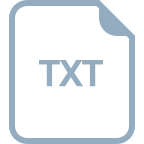
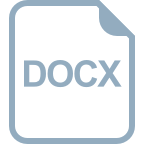
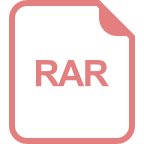
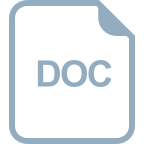
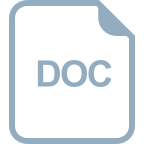
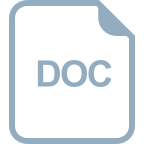
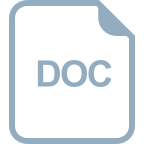
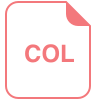




