请用C语言帮我写一个OpenGL创建八个不一样颜色面的八面体并加入旋转动画的代码
时间: 2023-06-04 17:08:28 浏览: 131
Sure, here is the code:
```c
#include <stdio.h>
#include <stdlib.h>
#include <GL/glut.h>
GLfloat vertices[][3] = {
{-1.0,-1.0,-1.0}, {1.0,-1.0,-1.0},
{1.0,1.0,-1.0}, {-1.0,1.0,-1.0},
{-1.0,-1.0,1.0}, {1.0,-1.0,1.0},
{1.0,1.0,1.0}, {-1.0,1.0,1.0}
};
GLfloat colors[][3] = {
{1.0, 0.0, 0.0}, {1.0, 1.0, 0.0},
{0.0, 1.0, 0.0}, {0.0, 0.0, 1.0},
{1.0, 0.0, 1.0}, {1.0, 1.0, 1.0},
{0.0, 1.0, 1.0}, {0.5, 0.5, 0.5}
};
static GLfloat theta[] = {0.0,0.0,0.0};
static GLint axis = 2;
static GLdouble viewer[]= {0.0, 0.0, 5.0};
static GLuint polyList;
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glLoadIdentity();
gluLookAt(viewer[0],viewer[1],viewer[2],0.0,0.0,0.0,0.0,1.0,0.0);
glRotatef(theta[0], 1.0, 0.0, 0.0);
glRotatef(theta[1], 0.0, 1.0, 0.0);
glRotatef(theta[2], 0.0, 0.0, 1.0);
glCallList(polyList);
glutSwapBuffers();
}
void spinCube()
{
theta[axis] += 1.0;
if(theta[axis] > 360.0) theta[axis] -= 360.0;
glutPostRedisplay();
}
void myKeyboard(unsigned char key, int x, int y)
{
if(key == 'x') viewer[0]-= 1.0;
if(key == 'X') viewer[0]+= 1.0;
if(key == 'y') viewer[1]-= 1.0;
if(key == 'Y') viewer[1]+= 1.0;
if(key == 'z') viewer[2]-= 1.0;
if(key == 'Z') viewer[2]+= 1.0;
glutPostRedisplay();
}
void myMouse(int btn, int state, int x, int y)
{
if(btn == GLUT_LEFT_BUTTON && state == GLUT_DOWN) axis = 0;
if(btn == GLUT_MIDDLE_BUTTON && state == GLUT_DOWN) axis = 1;
if(btn == GLUT_RIGHT_BUTTON && state == GLUT_DOWN) axis = 2;
}
void poly()
{
polyList = glGenLists(1);
glNewList(polyList, GL_COMPILE);
glBegin(GL_POLYGON);
glColor3fv(colors[0]);
glVertex3fv(vertices[0]);
glColor3fv(colors[1]);
glVertex3fv(vertices[1]);
glColor3fv(colors[2]);
glVertex3fv(vertices[2]);
glColor3fv(colors[3]);
glVertex3fv(vertices[3]);
glEnd();
glBegin(GL_POLYGON);
glColor3fv(colors[0]);
glVertex3fv(vertices[0]);
glColor3fv(colors[3]);
glVertex3fv(vertices[3]);
glColor3fv(colors[4]);
glVertex3fv(vertices[4]);
glColor3fv(colors[5]);
glVertex3fv(vertices[5]);
glEnd();
glBegin(GL_POLYGON);
glColor3fv(colors[5]);
glVertex3fv(vertices[5]);
glColor3fv(colors[4]);
glVertex3fv(vertices[4]);
glColor3fv(colors[7]);
glVertex3fv(vertices[7]);
glColor3fv(colors[6]);
glVertex3fv(vertices[6]);
glEnd();
glBegin(GL_POLYGON);
glColor3fv(colors[1]);
glVertex3fv(vertices[1]);
glColor3fv(colors[2]);
glVertex3fv(vertices[2]);
glColor3fv(colors[7]);
glVertex3fv(vertices[7]);
glColor3fv(colors[6]);
glVertex3fv(vertices[6]);
glEnd();
glBegin(GL_POLYGON);
glColor3fv(colors[0]);
glVertex3fv(vertices[3]);
glColor3fv(colors[4]);
glVertex3fv(vertices[4]);
glColor3fv(colors[7]);
glVertex3fv(vertices[7]);
glColor3fv(colors[2]);
glVertex3fv(vertices[2]);
glEnd();
glEndList();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_DEPTH);
glutInitWindowSize(500, 500);
glutInitWindowPosition(100, 100);
glutCreateWindow("Eight Colored Polyhedron");
glutKeyboardFunc(myKeyboard);
glutMouseFunc(myMouse);
glutDisplayFunc(display);
glutIdleFunc(spinCube);
glEnable(GL_DEPTH_TEST);
glClearColor(1.0, 1.0, 1.0, 0.0);
poly();
glutMainLoop();
return 0;
}
```
这是一个使用 OpenGL 和 GLUT 库创建八面体并加入旋转动画的代码。八面体的每个面都有不同的颜色。欢迎尝试运行代码,并在需要时进行修改和调整。
相关推荐
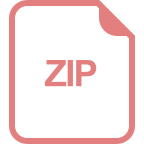
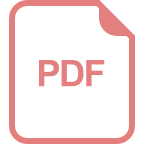














