如何修改pythonSVM 模型对象中的coef和intercept属性
时间: 2024-05-05 22:18:12 浏览: 6
您可以使用以下代码修改Python SVM模型对象中的coef和intercept属性:
```
# 导入相关库和模型
from sklearn import svm
import numpy as np
# 创建SVM模型
X = np.array([[0, 0], [1, 1]])
y = np.array([0, 1])
clf = svm.SVC(kernel='linear')
clf.fit(X, y)
# 打印原来的coef和intercept属性值
print("Original coef and intercept values:")
print(clf.coef_)
print(clf.intercept_)
# 按需修改coef和intercept属性值
new_coef = np.array([[0.5, 0.5]])
new_intercept = np.array([0.2])
# 设置新的属性值
clf.coef_ = new_coef
clf.intercept_ = new_intercept
# 打印修改后的coef和intercept属性值
print("Modified coef and intercept values:")
print(clf.coef_)
print(clf.intercept_)
```
您可以将这个代码片段放入您的项目中,然后按照您的需求修改coef和intercept属性,就可以达到修改模型对象的目的。
相关问题
python svm划分超平面
在Python中,可以使用scikit-learn库来实现SVM模型的训练和预测,同时也可以得到SVM模型的划分超平面。
以下是一个简单的示例代码,使用scikit-learn库中的SVM模型对Iris数据集进行分类,并绘制出SVM模型的划分超平面。
```python
from sklearn import datasets, svm
import matplotlib.pyplot as plt
import numpy as np
# 加载Iris数据集
iris = datasets.load_iris()
X = iris.data[:, :2]
y = iris.target
# 定义SVM模型
clf = svm.SVC(kernel='linear', C=1.0)
# 训练SVM模型
clf.fit(X, y)
# 绘制数据点
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.Set1)
# 绘制划分超平面
w = clf.coef_[0]
a = -w[0] / w[1]
xx = np.linspace(X[:, 0].min() - 1, X[:, 0].max() + 1, 100)
yy = a * xx - (clf.intercept_[0]) / w[1]
plt.plot(xx, yy, 'k-')
plt.xlabel('Sepal length')
plt.ylabel('Sepal width')
plt.show()
```
代码中使用了线性核函数(kernel='linear')的SVM模型,C参数默认为1.0。训练完成后,使用coef_和intercept_属性获取划分超平面的系数和截距,并绘制出划分超平面。
注意:以上代码仅为示例,实际应用中需要根据具体问题进行调整和优化。
python SVC intercept
在Python中,SVC类是scikit-learn库中用于支持向量机的分类算法的一个实现。它的参数包括C、kernel、degree、gamma、coef0等等。
SVC类中的属性intercept_用于表示决策函数中的常数项。可以通过调用fit方法拟合模型后,通过访问intercept_属性来获取这个常数项的值。
例如,在给定一个数据集和标签后,我们可以使用SVC对象进行二分类并拟合模型。然后,我们可以通过访问clf对象的intercept_属性来获取决策函数中的常数项。
所以,如果你想获得python SVC中的intercept,请通过访问SVC对象的intercept_属性来获取。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [Python scikit-learn 模块svc方法的参数解释](https://blog.csdn.net/AQ_cainiao_AQ/article/details/76025601)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [Python 线性 SVM 可视化](https://blog.csdn.net/qq_55745968/article/details/125668418)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
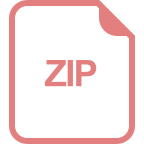












