opencv曲线拟合c++
时间: 2023-10-03 11:10:59 浏览: 100
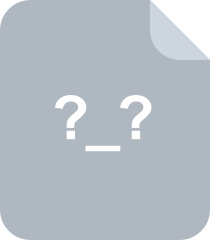
OpenCV中最小二乘曲线拟合C程序

以下是使用OpenCV进行曲线拟合的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 生成随机数据
Mat x(100, 1, CV_32FC1);
Mat y(100, 1, CV_32FC1);
randn(x, 0, 1);
randn(y, 0, 1);
// 定义多项式拟合的阶数
int n = 3;
// 拟合曲线
Mat A(x.rows, n + 1, CV_32FC1);
for (int i = 0; i < x.rows; i++)
{
float* Ai = A.ptr<float>(i);
float xi = x.at<float>(i);
for (int j = 0; j <= n; j++)
{
Ai[j] = pow(xi, j);
}
}
Mat coeffs;
solve(A, y, coeffs, DECOMP_QR);
// 绘制原始数据点
Mat img(300, 300, CV_8UC3, Scalar(255, 255, 255));
for (int i = 0; i < x.rows; i++)
{
Point pt(x.at<float>(i)*100 + 150, -y.at<float>(i)*100 + 150);
circle(img, pt, 2, Scalar(0, 0, 255), -1);
}
// 绘制拟合曲线
float* coeff = coeffs.ptr<float>();
for (int i = 0; i < img.cols; i++)
{
float x = (i - 150) / 100.0f;
float y = 0;
for (int j = 0; j <= n; j++)
{
y += coeff[j] * pow(x, j);
}
Point pt(i, -y*100 + 150);
circle(img, pt, 1, Scalar(0, 255, 0), -1);
}
// 显示图像
imshow("Curve Fitting", img);
waitKey();
return 0;
}
```
此代码生成100个随机数据点,然后使用3次多项式进行拟合,并绘制原始数据点和拟合曲线的图像。
阅读全文
相关推荐
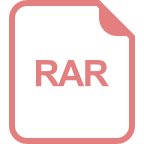
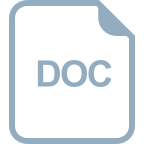















