class SelfAttention(nn.Module): def __init__(self, in_channels, reduction=4): super(SelfAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool1d(1) print("in_channels:", in_channels) print("reduction:", reduction) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() print("x.shape=", x.shape) # 查看每次SA模块输出的张量的形状,重点是c“通道”的维度 y = self.avg_pool(x) print("y.shape=", y.shape) # 查看每次经过avg_Pool操作后的y张量的变化 y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x)该模块中self.avg_pool = nn.AdaptiveAvgPool1d(1)语句是什么操作,有什么作用
时间: 2023-12-13 13:06:00 浏览: 184
该语句是对输入张量进行自适应平均池化操作,将每个通道内的特征图降为一个数,即将形状为 (b,c,n) 的输入张量 x 通过该语句转换为形状为 (b,c,1) 的输出张量 y。该操作的作用是从每个通道内提取出最具有代表性的特征,便于后续的特征处理。在该模块中,经过自注意力模块的处理后,最终的输出张量形状与输入张量相同,但是每个通道内的特征已经被加权处理过,有利于提高模型的特征表达能力。
相关问题
SelfAttention(channel_out)该语句的作用是什么,我知道这个类的定义是:class SelfAttention(nn.Module): def __init__(self, in_channels, reduction=4): super(SelfAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool1d(1) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x)
这行代码的作用是用一个全连接层(fc1)来对输入的特征进行降维,然后通过ReLU激活函数进行非线性变换,再用另一个全连接层(fc2)将特征恢复到原来的维度。最后,通过Sigmoid函数将这个特征变换应用到原始特征向量上,以产生表示哪些部分应该被突出的向量,这个过程就是所谓的"自注意力"机制。因此,类SelfAttention的作用是实现一个自注意力机制,用于对输入数据进行特征提取和加权。
这是类的定义:class SelfAttention(nn.Module): def __init__(self, in_channels, reduction=4): super(SelfAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool1d(1) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x);而这是使用该类时的语句SelfAttention(channel_out),所以该语句是将channel_out传递给类中的in_channels吗?
是的,你的理解是正确的。在实例化`SelfAttention`类时,你需要传递一个`in_channels`参数,它将被用作输入张量`x`的通道数。在你的代码中,你使用`channel_out`来实例化`SelfAttention`类,因此`channel_out`将被传递给`in_channels`。在`forward`函数中,`x`表示输入张量,其大小为(b, c, n),其中b是批次大小,c是通道数,n是序列长度。
阅读全文
相关推荐
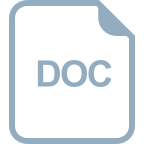
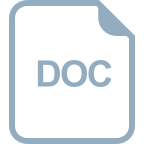
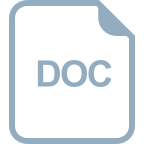









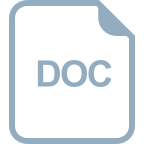
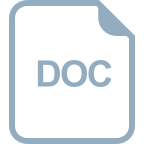
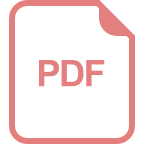
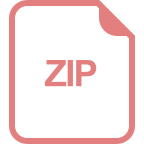