使用pytorch实现文本和图片的cross attention
时间: 2023-08-26 21:06:48 浏览: 314
首先,我们需要定义一个自定义的CrossModalAttention层,它接收两个输入:文本和图片,然后进行交叉注意力的计算。
```python
import torch
import torch.nn as nn
class CrossModalAttention(nn.Module):
def __init__(self, text_dim, img_dim, hidden_dim):
super(CrossModalAttention, self).__init__()
self.text_dim = text_dim
self.img_dim = img_dim
self.hidden_dim = hidden_dim
self.w_text = nn.Linear(text_dim, hidden_dim)
self.w_img = nn.Linear(img_dim, hidden_dim)
self.softmax = nn.Softmax(dim=1)
def forward(self, text, img):
text_proj = self.w_text(text)
img_proj = self.w_img(img)
scores = torch.matmul(text_proj, img_proj.transpose(1, 2))
text_att = self.softmax(scores)
img_att = self.softmax(scores.transpose(1, 2))
text_weighted = torch.matmul(text_att, img)
img_weighted = torch.matmul(img_att, text)
return text_weighted, img_weighted
```
接下来,我们可以使用这个自定义层来构建一个简单的跨模态交叉注意力模型。
我们使用了一个文本嵌入层和一个图片嵌入层来将输入文本和图片转换为向量表示。然后,我们使用CrossModalAttention层计算交叉注意力,并将结果传递到后续的全连接层进行分类。
```python
import torchvision.models as models
class CrossModalModel(nn.Module):
def __init__(self, text_dim, img_dim, hidden_dim, num_classes):
super(CrossModalModel, self).__init__()
self.text_dim = text_dim
self.img_dim = img_dim
self.hidden_dim = hidden_dim
self.num_classes = num_classes
self.text_embed = nn.Embedding(text_dim, hidden_dim)
self.img_embed = models.resnet18(pretrained=True)
self.img_embed.fc = nn.Linear(512, img_dim)
self.crossmodal_att = CrossModalAttention(text_dim=hidden_dim, img_dim=img_dim, hidden_dim=hidden_dim)
self.fc = nn.Linear(hidden_dim*2, num_classes)
def forward(self, text, img):
text_embedded = self.text_embed(text)
img_embedded = self.img_embed(img)
text_weighted, img_weighted = self.crossmodal_att(text_embedded, img_embedded)
x = torch.cat([text_weighted, img_weighted], dim=1)
out = self.fc(x)
return out
```
现在我们可以使用这个模型来训练文本和图片分类任务了。我们只需要将文本和图片作为输入,然后计算交叉注意力,并将结果传递到分类器中。
```python
import torch.optim as optim
from torch.utils.data import DataLoader
# define your dataset for text and image
dataset = MyDataset(text_data, img_data, labels)
# define your dataloader
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
# define your cross-modal model
model = CrossModalModel(text_dim=100, img_dim=512, hidden_dim=256, num_classes=10)
# define your loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# train the model
for epoch in range(num_epochs):
for text, img, labels in dataloader:
optimizer.zero_grad()
outputs = model(text, img)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
阅读全文
相关推荐
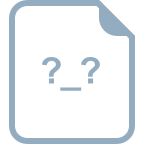
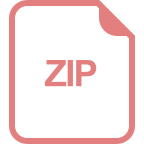
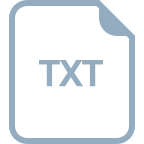
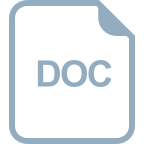
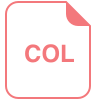
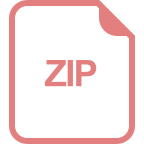
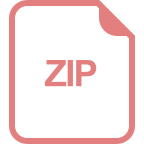
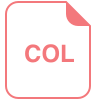
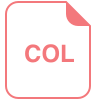
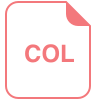
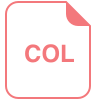
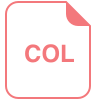
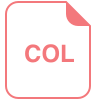
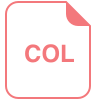
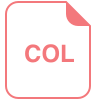
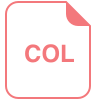


