python 中缀表达式转后缀
时间: 2023-10-11 10:11:33 浏览: 120
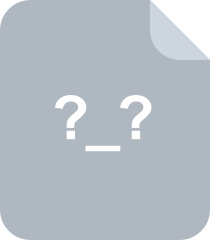
中缀转后缀表达式
在 Python 中,可以通过栈来实现中缀表达式转后缀表达式的过程。具体实现如下:
```python
def infix_to_postfix(expr):
# 定义运算符优先级
priority = {'+': 1, '-': 1, '*': 2, '/': 2, '^': 3}
# 定义一个栈和一个空的后缀表达式列表
stack = []
postfix = []
# 遍历中缀表达式的每个字符
for char in expr:
# 如果是数字或字母,直接加入后缀表达式列表
if char.isdigit() or char.isalpha():
postfix.append(char)
# 如果是左括号,压入栈中
elif char == '(':
stack.append(char)
# 如果是右括号,弹出栈中的运算符,直到遇到左括号,并将这些运算符加入后缀表达式列表
elif char == ')':
while stack[-1] != '(':
postfix.append(stack.pop())
stack.pop() # 弹出左括号
# 如果是运算符,弹出栈中优先级大于等于该运算符的所有运算符,并将它们加入后缀表达式列表
elif char in priority:
while stack and stack[-1] != '(' and priority[char] <= priority[stack[-1]]:
postfix.append(stack.pop())
stack.append(char)
# 将栈中剩余的运算符加入后缀表达式列表
while stack:
postfix.append(stack.pop())
# 将后缀表达式列表转为字符串并返回
return ''.join(postfix)
```
例子:
```python
expr = "a+b*c-d/e"
print(infix_to_postfix(expr)) # 输出:abc*+de/-
```
这里将中缀表达式 "a+b*c-d/e" 转为了后缀表达式 "abc*+de/-"。
阅读全文
相关推荐
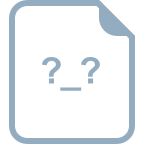


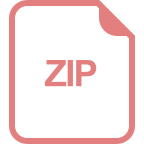



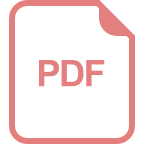
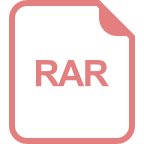
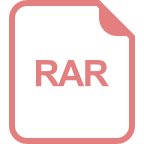
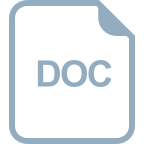
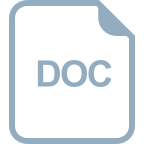
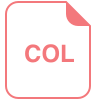



