java代码写一个八皇后
时间: 2024-05-03 22:22:56 浏览: 89
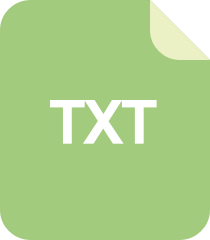
八皇后算法的java实现源代码
下面是一个简单的Java代码实现八皇后问题:
```
public class EightQueens {
private static final int SIZE = 8;
private int[] queens = new int[SIZE];
private int count = 0;
public void solve() {
placeQueen(0);
System.out.println("Total solutions: " + count);
}
private void placeQueen(int row) {
if (row == SIZE) {
printSolution();
count++;
} else {
for (int col = 0; col < SIZE; col++) {
queens[row] = col;
if (isValid(row, col)) {
placeQueen(row + 1);
}
}
}
}
private boolean isValid(int row, int col) {
for (int i = 0; i < row; i++) {
int diff = Math.abs(queens[i] - col);
if (diff == 0 || diff == row - i) {
return false;
}
}
return true;
}
private void printSolution() {
for (int row = 0; row < SIZE; row++) {
for (int col = 0; col < SIZE; col++) {
if (queens[row] == col) {
System.out.print("Q ");
} else {
System.out.print(". ");
}
}
System.out.println();
}
System.out.println();
}
public static void main(String[] args) {
EightQueens eq = new EightQueens();
eq.solve();
}
}
```
该程序使用回溯法解决八皇后问题。在placeQueen方法中,先放置第0行皇后的位置,然后递归地放置第1行、第2行……直到第7行皇后的位置。在放置皇后时,使用isValid方法来检查当前位置是否与之前的皇后位置冲突。如果当前位置合法,则继续递归放置下一行皇后。如果在第7行放置了皇后,则说明找到了一个解,打印出来并增加计数器。最后,输出所有解的总数。
阅读全文
相关推荐
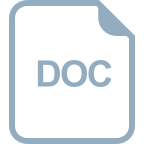
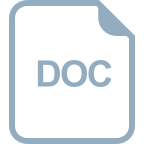
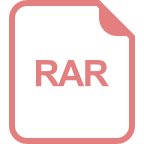
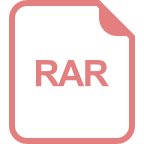









