ffmpeg推流初始化代码
时间: 2023-09-29 18:11:23 浏览: 56
以下是使用FFmpeg库进行推流的初始化代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <errno.h>
#include <signal.h>
#include <sys/time.h>
#include <sys/select.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <pthread.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
#include <libavutil/pixdesc.h>
#include <libavutil/time.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[])
{
AVFormatContext *fmt_ctx = NULL;
AVOutputFormat *out_fmt = NULL;
AVStream *video_st = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
int ret;
// 初始化FFmpeg库
av_register_all();
avformat_network_init();
// 打开输出文件
ret = avformat_alloc_output_context2(&fmt_ctx, NULL, "flv", "rtmp://127.0.0.1/live/stream");
if (ret < 0) {
printf("Could not allocate output format context\n");
return -1;
}
out_fmt = fmt_ctx->oformat;
// 添加音视频流
video_st = avformat_new_stream(fmt_ctx, NULL);
if (!video_st) {
printf("Could not create video stream\n");
return -1;
}
codec_ctx = video_st->codec;
// 设置编码器参数
codec_ctx->codec_id = out_fmt->video_codec;
codec_ctx->codec_type = AVMEDIA_TYPE_VIDEO;
codec_ctx->width = 640;
codec_ctx->height = 480;
codec_ctx->time_base.num = 1;
codec_ctx->time_base.den = 25;
codec = avcodec_find_encoder(codec_ctx->codec_id);
if (!codec) {
printf("Could not find encoder\n");
return -1;
}
// 打开编码器
ret = avcodec_open2(codec_ctx, codec, NULL);
if (ret < 0) {
printf("Could not open encoder\n");
return -1;
}
// 打开输出流
ret = avio_open(&fmt_ctx->pb, fmt_ctx->filename, AVIO_FLAG_WRITE);
if (ret < 0) {
printf("Could not open output file\n");
return -1;
}
// 写文件头
ret = avformat_write_header(fmt_ctx, NULL);
if (ret < 0) {
printf("Error occurred when writing file header\n");
return -1;
}
// 推流
AVFrame *frame = av_frame_alloc();
AVPacket pkt;
int i;
for (i = 0; i < 100; i++) {
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
// 生成测试图像
uint8_t *data[1];
int linesize[1];
int height = codec_ctx->height;
int width = codec_ctx->width;
data[0] = (uint8_t*)malloc(width * height * 3);
int y;
for (y = 0; y < height; y++) {
memset(data[0] + y * width * 3, y + i * 3, width * 3);
}
linesize[0] = width * 3;
// 填充AVFrame
frame->format = codec_ctx->pix_fmt;
frame->width = codec_ctx->width;
frame->height = codec_ctx->height;
av_image_fill_arrays(frame->data, frame->linesize, data[0], codec_ctx->pix_fmt, codec_ctx->width, codec_ctx->height, 1);
// 编码并发送数据
int got_picture = 0;
ret = avcodec_encode_video2(codec_ctx, &pkt, frame, &got_picture);
if (ret < 0) {
printf("Error encoding video frame\n");
return -1;
}
if (got_picture) {
pkt.stream_index = video_st->index;
ret = av_write_frame(fmt_ctx, &pkt);
if (ret < 0) {
printf("Error writing video frame\n");
return -1;
}
}
av_free_packet(&pkt);
av_freep(&data[0]);
}
// 写文件尾
av_write_trailer(fmt_ctx);
// 释放资源
avio_close(fmt_ctx->pb);
avcodec_close(codec_ctx);
avformat_free_context(fmt_ctx);
av_frame_free(&frame);
return 0;
}
```
这段代码将以FLV格式将一组测试图像推流到`rtmp://127.0.0.1/live/stream`位置。你可以根据需要修改推流地址等参数。
相关推荐
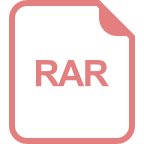














