Q = tf.layers.dense(inputs=Q, units=units, activation=tf.nn.relu) AttributeError: module 'tensorflow' has no attribute 'layers'
时间: 2023-12-17 14:02:19 浏览: 120
这个错误通常是因为使用的 TensorFlow 版本太低导致的。在较旧的 TensorFlow 版本中,`layers` 模块不是 TensorFlow 的一部分,而是一个单独的模块。
为了解决这个问题,您可以升级 TensorFlow 到最新版本。如果您已经使用了最新版本的 TensorFlow,那么您的代码可能存在其他问题,需要进一步调试。
相关问题
AttributeError: module 'tensorflow.keras.layers' has no attribute 'transformer'这个问题如何解决,添加transformer层
如果你想手动添加transformer层,可以参考以下代码实现:
```python
import tensorflow as tf
class MultiHeadSelfAttention(tf.keras.layers.Layer):
def __init__(self, embed_dim, num_heads, **kwargs):
super().__init__(**kwargs)
self.embed_dim = embed_dim
self.num_heads = num_heads
if embed_dim % num_heads != 0:
raise ValueError(
f"embedding dimension = {embed_dim} should be divisible by number of heads = {num_heads}"
)
self.projection_dim = embed_dim // num_heads
self.query_dense = tf.keras.layers.Dense(embed_dim)
self.key_dense = tf.keras.layers.Dense(embed_dim)
self.value_dense = tf.keras.layers.Dense(embed_dim)
self.combine_heads = tf.keras.layers.Dense(embed_dim)
def attention(self, query, key, value):
score = tf.matmul(query, key, transpose_b=True)
dim_key = tf.cast(tf.shape(key)[-1], tf.float32)
scaled_score = score / tf.math.sqrt(dim_key)
weights = tf.nn.softmax(scaled_score, axis=-1)
output = tf.matmul(weights, value)
return output, weights
def separate_heads(self, x, batch_size):
x = tf.reshape(x, (batch_size, -1, self.num_heads, self.projection_dim))
return tf.transpose(x, perm=[0, 2, 1, 3])
def call(self, inputs):
batch_size = tf.shape(inputs)[0]
query = self.query_dense(inputs)
key = self.key_dense(inputs)
value = self.value_dense(inputs)
query = self.separate_heads(query, batch_size)
key = self.separate_heads(key, batch_size)
value = self.separate_heads(value, batch_size)
attention, weights = self.attention(query, key, value)
attention = tf.transpose(attention, perm=[0, 2, 1, 3])
concat_attention = tf.reshape(attention, (batch_size, -1, self.embed_dim))
output = self.combine_heads(concat_attention)
return output
class TransformerBlock(tf.keras.layers.Layer):
def __init__(self, embed_dim, num_heads, ff_dim, rate=0.1, **kwargs):
super().__init__(**kwargs)
self.att = MultiHeadSelfAttention(embed_dim, num_heads)
self.ffn = tf.keras.Sequential(
[tf.keras.layers.Dense(ff_dim, activation="relu"), tf.keras.layers.Dense(embed_dim),]
)
self.layernorm1 = tf.keras.layers.LayerNormalization(epsilon=1e-6)
self.layernorm2 = tf.keras.layers.LayerNormalization(epsilon=1e-6)
self.dropout1 = tf.keras.layers.Dropout(rate)
self.dropout2 = tf.keras.layers.Dropout(rate)
def call(self, inputs, training):
attn_output = self.att(inputs)
attn_output = self.dropout1(attn_output, training=training)
out1 = self.layernorm1(inputs + attn_output)
ffn_output = self.ffn(out1)
ffn_output = self.dropout2(ffn_output, training=training)
return self.layernorm2(out1 + ffn_output)
class TokenAndPositionEmbedding(tf.keras.layers.Layer):
def __init__(self, maxlen, vocab_size, embed_dim, **kwargs):
super().__init__(**kwargs)
self.token_emb = tf.keras.layers.Embedding(input_dim=vocab_size, output_dim=embed_dim)
self.pos_emb = tf.keras.layers.Embedding(input_dim=maxlen, output_dim=embed_dim)
def call(self, x):
maxlen = tf.shape(x)[-1]
positions = tf.range(start=0, limit=maxlen, delta=1)
positions = self.pos_emb(positions)
x = self.token_emb(x)
return x + positions
class Transformer(tf.keras.Model):
def __init__(self, vocab_size, maxlen, embed_dim, num_heads, ff_dim, num_layers=4, **kwargs):
super().__init__(**kwargs)
self.embedding = TokenAndPositionEmbedding(maxlen, vocab_size, embed_dim)
self.transformer_blocks = [TransformerBlock(embed_dim, num_heads, ff_dim) for _ in range(num_layers)]
self.out = tf.keras.layers.Dense(vocab_size, activation="softmax")
def call(self, inputs, training):
x = self.embedding(inputs)
for block in self.transformer_blocks:
x = block(x, training)
x = self.out(x)
return x
```
这里实现了一个基本的Transformer模型,包括多头自注意力机制(MultiHeadSelfAttention)、Transformer块(TransformerBlock)、位置编码嵌入层(TokenAndPositionEmbedding)和Transformer模型(Transformer)。你可以根据需要调整其中的参数来满足你的需求。
AttributeError: module 'tensorflow.compat.v1' has no attribute 'contrib'
This error occurs when you try to use the "contrib" module from TensorFlow v1 in TensorFlow v2. The "contrib" module was removed from TensorFlow v2, so you will need to update your code to use the new modules and functions available in TensorFlow v2.
Here are a few steps you can take to resolve this error:
1. Update your code to use the new modules and functions available in TensorFlow v2. You can find the equivalent functions in the new API documentation.
2. If you need to use the "contrib" module, you can install TensorFlow v1.x and use it in a separate environment.
3. If you are using a pre-trained model that was trained on TensorFlow v1, you can try using the "tensorflow.compat.v1" module to run the model in TensorFlow v2.
For example, if you have a line of code like this:
```python
from tensorflow.contrib import layers
```
You can update it to use the new API like this:
```python
import tensorflow as tf
from tensorflow.keras import layers
# Use the new API instead of the "contrib" module
x = layers.Dense(128, activation='relu')(inputs)
```
Or, you can use the "tensorflow.compat.v1" module like this:
```python
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
# Use the "contrib" module in TensorFlow v1.x
x = tf.contrib.layers.fully_connected(inputs, 128, activation_fn=tf.nn.relu)
```
阅读全文
相关推荐
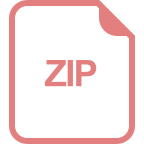
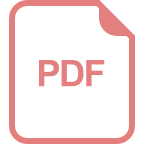
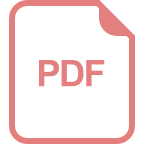
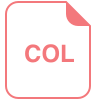
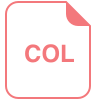








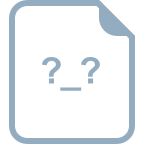
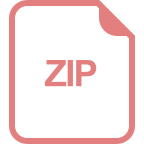
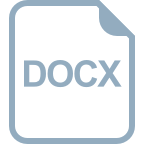
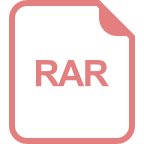