opencv 检测图片中的圆形,输出圆的坐标和半径 c++ 代码
时间: 2023-06-15 15:06:57 浏览: 188
可以使用OpenCV中的Hough圆检测算法来检测图片中的圆形。以下是一个简单的C++代码示例,用于检测圆形并输出圆的坐标和半径:
```c++
#include "opencv2/highgui.hpp"
#include "opencv2/imgproc.hpp"
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
if (argc != 2)
{
cout << "Usage: " << argv[0] << " <image_path>" << endl;
return -1;
}
// Read image
Mat src = imread(argv[1], IMREAD_GRAYSCALE);
if (src.empty())
{
cout << "Failed to read image" << endl;
return -1;
}
// Apply Gaussian blur to reduce noise
Mat blurred;
GaussianBlur(src, blurred, Size(5, 5), 0);
// Apply Hough circle detection
vector<Vec3f> circles;
HoughCircles(blurred, circles, HOUGH_GRADIENT, 1, src.rows / 8, 200, 100, 0, 0);
// Draw detected circles
Mat output = src.clone();
for (size_t i = 0; i < circles.size(); i++)
{
Point center(cvRound(circles[i][0]), cvRound(circles[i][1]));
int radius = cvRound(circles[i][2]);
circle(output, center, radius, Scalar(0, 0, 255), 2, LINE_AA);
cout << "Circle " << i << ": center=(" << center.x << "," << center.y << "), radius=" << radius << endl;
}
// Show output
namedWindow("Output", WINDOW_NORMAL);
imshow("Output", output);
waitKey(0);
return 0;
}
```
该代码首先读取指定路径的图像,然后对其进行高斯模糊以减少噪声。接下来,它使用Hough圆检测算法来检测图像中的圆形,将检测到的圆形绘制到输出图像上,并输出每个圆的坐标和半径。最后,它显示输出图像并等待用户按下任意键。
相关推荐
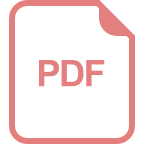














