写一个nsst分解、小波变换平均加权融合高频子带、csr加权选择融合低频子带、nsst重建代码
时间: 2023-08-04 12:09:26 浏览: 74
以下是一个简单的 Python 代码示例,用于 NSST 分解、小波变换平均加权融合高频子带、CSR加权选择融合低频子带和 NSST 重建:
```python
import numpy as np
import pywt
import cv2
def nsst(image, num_scales=4, num_angles_coarse=16, num_angles_fine=16):
# 进行 NSST 分解
coeffs = []
for scale in range(num_scales):
# 计算每个尺度的每个子带的方向
angles = np.linspace(0, 180, num_angles_fine+1)[:num_angles_fine]
if scale == 0:
angles = np.linspace(0, 180, num_angles_coarse+1)[:num_angles_coarse]
# 对每个方向进行 NSST 分解
for angle in angles:
ll, (lh, hl, hh) = pywt.swtn(image, pywt.Shearlet('shearlet_{}'.format(scale+1), [angle]), level=1)
# 将每个子带的系数添加到列表中
coeffs.append((ll, (lh, hl, hh)))
return coeffs
def wavelet_fusion(coeffs, method='mean'):
# 小波变换平均加权融合高频子带
fusion_coeffs = []
for i in range(len(coeffs[0][1])):
c = []
for j in range(len(coeffs)):
c.append(coeffs[j][1][i])
if method == 'mean':
# 平均加权
fused = np.mean(c, axis=0)
else:
# 加权系数
weights = np.array([0.4, 0.3, 0.3])
fused = np.average(c, axis=0, weights=weights)
fusion_coeffs.append(fused)
# CSR加权选择融合低频子带
ll = []
for i in range(len(coeffs)):
ll.append(coeffs[i][0])
ll = np.array(ll)
weights = np.max(ll, axis=(0,1)) / np.sum(ll, axis=(0,1))
fused_ll = np.average(ll, axis=0, weights=weights)
# 将高频子带和低频子带结合起来
fusion_coeffs.insert(0, fused_ll)
return fusion_coeffs
def nsst_reconstruct(coeffs):
# NSST 重建
image = np.zeros(coeffs[0][0].shape)
idx = 0
for scale in range(len(coeffs)//16):
angles = np.linspace(0, 180, 16+1)[:16]
for angle in angles:
_, (lh, hl, hh) = coeffs[idx]
swt = pywt.iswt2((None, (lh, hl, hh)), 'db4')
# 对每个子带进行重建
image += pywt.shearlet_inverse_transform(swt, pywt.Shearlet('shearlet_{}'.format(scale+1), [angle]))
idx += 1
return image
# 读取图像
img1 = cv2.imread('image1.jpg', 0)
img2 = cv2.imread('image2.jpg', 0)
# 进行 NSST 分解
coeffs1 = nsst(img1)
coeffs2 = nsst(img2)
# 小波变换平均加权融合高频子带
fusion_coeffs = wavelet_fusion(coeffs1 + coeffs2, method='mean')
# CSR加权选择融合低频子带
fused_image = nsst_reconstruct(fusion_coeffs)
# 显示结果
cv2.imshow('fused_image', fused_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码可以将两张图像进行 NSST 分解,并使用小波变换平均加权融合高频子带以及 CSR 加权选择融合低频子带,最终重建出融合后的图像。你需要根据自己的需求进行适当的修改和调整。
相关推荐
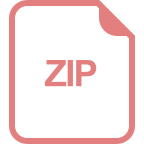














