template <class x> struct template_type{ using template_type_t = x; };template<> struct template_type<bool> { };是什么意思
时间: 2024-04-28 15:23:50 浏览: 9
这一个 C++ 的模板类,其中定义了一个模板参数为类型 x 的结构体 template_type。其中使用了一个类型别名 template_type_t,用来表示模板参数 x 的类型。
在模板参数为 bool 的情况下,定义了一个特化版本的 template_type,但是没有为其定义 template_type_t 的类型别名,因此无法在 bool 类型的情况下使用 template_type_t。
相关问题
template <class x> struct template_type{ using template_type_t = x; };template<> struct template_type<bool> : true_type { };
这段代码是上一个问题的扩展,增加了一个继承自 `true_type` 的模板特化版本。`true_type` 是一个 C++ 标准库中定义的类型,它是一个类模板,用于表示一个常量 boolean 值为 true 的类型。
在这个例子中,当模板参数为 `bool` 时,使用了模板特化,继承了 `true_type`,表示这个类型的值为 true。这个特化版本中,没有定义 `template_type_t`,因此在 `bool` 类型的情况下,`template_type_t` 仍然无法使用。
这个例子展示了模板特化的另一种用法,即可以通过继承某个类,来为某种类型提供额外的功能。在这个例子中,特化版本继承了 `true_type`,可以利用 `true_type` 提供的功能。
std::conditional的value_type用法
`std::conditional` 是一个模板类,它可以根据一个条件表达式的真假来决定类型的选择。它的语法如下:
```
template<bool B, class T, class F>
struct conditional;
```
其中,`B` 是一个布尔值,表示条件表达式的真假;`T` 和 `F` 分别是两个类型,当 `B` 为真时,`conditional` 的类型为 `T`,否则为 `F`。
`value_type` 不是 `std::conditional` 中的一个成员,但是可以用于表示 `T` 或 `F` 的类型中的元素类型。例如:
```c++
#include <iostream>
#include <type_traits>
#include <vector>
int main() {
using type1 = std::vector<int>::value_type; // int
using type2 = std::vector<double>::value_type; // double
using result_type1 = std::conditional<true, type1, type2>::type; // int
using result_type2 = std::conditional<false, type1, type2>::type; // double
std::cout << std::boolalpha;
std::cout << "result_type1 is int? " << std::is_same<result_type1, int>::value << std::endl;
std::cout << "result_type2 is double? " << std::is_same<result_type2, double>::value << std::endl;
return 0;
}
```
在上面的代码中,我们使用 `std::vector<int>` 和 `std::vector<double>` 分别表示 `type1` 和 `type2` 类型中的元素类型。然后,我们使用 `std::conditional` 来根据条件表达式的真假来选择元素类型。最后,我们使用 `std::is_same` 来判断 `result_type1` 和 `result_type2` 是否分别为 `int` 和 `double` 类型。
相关推荐
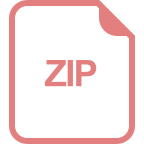
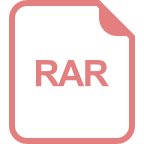
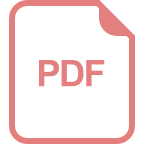













