编写一个mapreduce的成绩统计学生平均成绩,输入文件的格式为 学号:课程号:成绩分数,要求输出为学号:平均绩点GPA。绩点GPA计算方式按上海理工大学规定。Java
时间: 2024-02-19 14:59:47 浏览: 125
好的,这是一个典型的MapReduce程序,需要分为两个阶段:Map阶段和Reduce阶段。以下是Java代码实现:
Map阶段:
```
public static class Map extends Mapper<LongWritable, Text, Text, FloatWritable> {
private Text outputKey = new Text();
private FloatWritable outputValue = new FloatWritable();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(":");
if (fields.length != 3) {
return;
}
String studentId = fields[0];
String courseId = fields[1];
float score = Float.parseFloat(fields[2]);
// 计算绩点GPA
float gpa = 0;
if (score >= 90) {
gpa = 4.0f;
} else if (score >= 85) {
gpa = 3.7f;
} else if (score >= 82) {
gpa = 3.3f;
} else if (score >= 78) {
gpa = 3.0f;
} else if (score >= 75) {
gpa = 2.7f;
} else if (score >= 72) {
gpa = 2.3f;
} else if (score >= 68) {
gpa = 2.0f;
} else if (score >= 64) {
gpa = 1.5f;
} else if (score >= 60) {
gpa = 1.0f;
}
// 计算学号:课程号的组合作为Map输出的key
outputKey.set(studentId + ":" + courseId);
outputValue.set(gpa);
context.write(outputKey, outputValue);
}
}
```
Reduce阶段:
```
public static class Reduce extends Reducer<Text, FloatWritable, Text, FloatWritable> {
private FloatWritable outputValue = new FloatWritable();
public void reduce(Text key, Iterable<FloatWritable> values, Context context) throws IOException, InterruptedException {
float totalGPA = 0;
int count = 0;
for (FloatWritable value : values) {
totalGPA += value.get();
count++;
}
// 计算平均绩点GPA
float avgGPA = totalGPA / count;
// 输出学号:平均绩点GPA的组合作为Reduce输出的key
context.write(new Text(key.toString().split(":")[0]), new FloatWritable(avgGPA));
}
}
```
最后,在Driver类中设置Job的输入路径、输出路径、Mapper和Reducer,然后运行Job即可完成统计。
完整代码如下:
```
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.FloatWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class ScoreGPA {
public static class Map extends Mapper<LongWritable, Text, Text, FloatWritable> {
private Text outputKey = new Text();
private FloatWritable outputValue = new FloatWritable();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(":");
if (fields.length != 3) {
return;
}
String studentId = fields[0];
String courseId = fields[1];
float score = Float.parseFloat(fields[2]);
// 计算绩点GPA
float gpa = 0;
if (score >= 90) {
gpa = 4.0f;
} else if (score >= 85) {
gpa = 3.7f;
} else if (score >= 82) {
gpa = 3.3f;
} else if (score >= 78) {
gpa = 3.0f;
} else if (score >= 75) {
gpa = 2.7f;
} else if (score >= 72) {
gpa = 2.3f;
} else if (score >= 68) {
gpa = 2.0f;
} else if (score >= 64) {
gpa = 1.5f;
} else if (score >= 60) {
gpa = 1.0f;
}
// 计算学号:课程号的组合作为Map输出的key
outputKey.set(studentId + ":" + courseId);
outputValue.set(gpa);
context.write(outputKey, outputValue);
}
}
public static class Reduce extends Reducer<Text, FloatWritable, Text, FloatWritable> {
private FloatWritable outputValue = new FloatWritable();
public void reduce(Text key, Iterable<FloatWritable> values, Context context) throws IOException, InterruptedException {
float totalGPA = 0;
int count = 0;
for (FloatWritable value : values) {
totalGPA += value.get();
count++;
}
// 计算平均绩点GPA
float avgGPA = totalGPA / count;
// 输出学号:平均绩点GPA的组合作为Reduce输出的key
context.write(new Text(key.toString().split(":")[0]), new FloatWritable(avgGPA));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "ScoreGPA");
job.setJarByClass(ScoreGPA.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(FloatWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
阅读全文
相关推荐
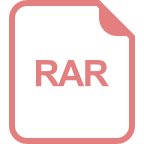
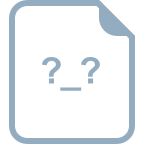
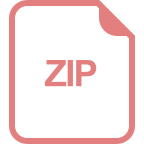
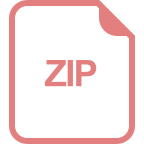
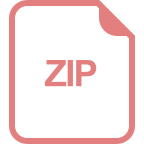
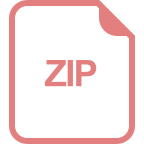
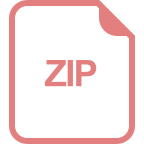
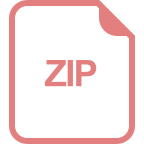
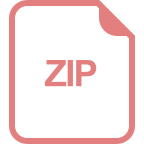
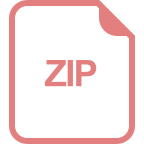
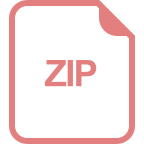
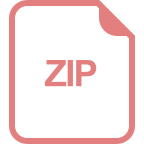
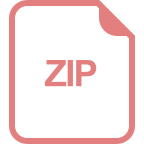
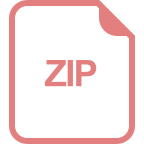
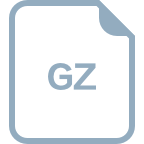