C++ 实现lvx点云格式转换pcd,不依赖第三方sdk,和运行示例
时间: 2024-01-07 22:04:20 浏览: 31
首先,需要了解lvx格式和pcd格式是什么。lvx格式是Livox激光雷达原始数据格式,包含了点云的XYZ、强度和角度等信息;pcd格式是点云库PCL使用的点云数据格式,包含了点云的XYZRGB、法向量、曲率等信息。
下面是C++实现lvx点云格式转换pcd的示例代码:
```cpp
#include <iostream>
#include <fstream>
#pragma pack(push, 1)
struct LivoxPoint {
float x;
float y;
float z;
uint8_t intensity;
uint16_t reflectivity;
uint16_t ambient;
uint16_t noise;
uint8_t tag;
uint8_t line_number;
uint16_t valid;
};
#pragma pack(pop)
const int kLvxFrameSize = 1206 * 4;
const int kLvxBlockSize = 100;
bool ConvertLvxToPcd(const std::string& lvx_file, const std::string& pcd_file) {
std::ifstream ifs(lvx_file, std::ios::binary);
if (!ifs) {
std::cerr << "Failed to open lvx file: " << lvx_file << std::endl;
return false;
}
std::ofstream ofs(pcd_file, std::ios::binary);
if (!ofs) {
std::cerr << "Failed to open pcd file: " << pcd_file << std::endl;
return false;
}
// Write pcd header
ofs << "# .PCD v0.7 - Point Cloud Data file format\n";
ofs << "VERSION 0.7\n";
ofs << "FIELDS x y z intensity\n";
ofs << "SIZE 4 4 4 1\n";
ofs << "TYPE F F F U\n";
ofs << "COUNT 1 1 1 1\n";
ofs << "WIDTH 0\n";
ofs << "HEIGHT 1\n";
ofs << "VIEWPOINT 0 0 0 1 0 0 0\n";
ofs << "POINTS 0\n";
ofs << "DATA ascii\n";
// Read lvx data
char lvx_frame[kLvxFrameSize];
LivoxPoint lvx_points[kLvxBlockSize];
int point_count = 0;
while (ifs.read(lvx_frame, kLvxFrameSize)) {
memcpy(lvx_points, lvx_frame, kLvxBlockSize * sizeof(LivoxPoint));
for (int i = 0; i < kLvxBlockSize; ++i) {
if (lvx_points[i].valid) {
float x = lvx_points[i].x / 1000.0f; // Convert from mm to m
float y = lvx_points[i].y / 1000.0f;
float z = lvx_points[i].z / 1000.0f;
uint8_t intensity = lvx_points[i].intensity;
ofs.write(reinterpret_cast<char*>(&x), sizeof(float));
ofs.write(reinterpret_cast<char*>(&y), sizeof(float));
ofs.write(reinterpret_cast<char*>(&z), sizeof(float));
ofs.write(reinterpret_cast<char*>(&intensity), sizeof(uint8_t));
++point_count;
}
}
}
// Update pcd header
ofs.seekp(0, std::ios::beg);
ofs << "# .PCD v0.7 - Point Cloud Data file format\n";
ofs << "VERSION 0.7\n";
ofs << "FIELDS x y z intensity\n";
ofs << "SIZE 4 4 4 1\n";
ofs << "TYPE F F F U\n";
ofs << "COUNT 1 1 1 1\n";
ofs << "WIDTH " << point_count << "\n";
ofs << "HEIGHT 1\n";
ofs << "VIEWPOINT 0 0 0 1 0 0 0\n";
ofs << "POINTS " << point_count << "\n";
ofs << "DATA binary\n";
return true;
}
int main(int argc, char* argv[]) {
if (argc != 3) {
std::cerr << "Usage: " << argv[0] << " lvx_file pcd_file" << std::endl;
return 1;
}
if (!ConvertLvxToPcd(argv[1], argv[2])) {
return 1;
}
return 0;
}
```
这段代码实现了将lvx格式的点云数据转换为pcd格式的点云数据,其中涉及到了文件读写、二进制数据处理和点云格式转换等知识点。需要注意的是,这段代码仅适用于Livox激光雷达的点云数据转换,其他类型的点云数据需要根据实际情况进行修改。
相关推荐










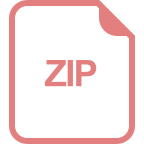
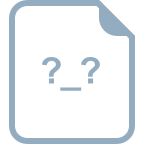
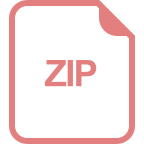
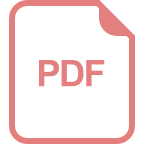