Long.parseLong(output.get(6))/(1024*1024) 将结果保留两位有效数字
时间: 2024-03-02 18:53:17 浏览: 102
可以使用 Java 中的 DecimalFormat 类来实现保留两位有效数字的功能,代码如下:
```
double result = Long.parseLong(output.get(6))/(1024*1024);
DecimalFormat decimalFormat = new DecimalFormat("#0.00");
String formattedResult = decimalFormat.format(result);
```
其中,`#0.00` 表示保留两位有效数字的格式。`format` 方法将会返回一个字符串类型的结果 `formattedResult`,即保留了两位有效数字的结果。
阅读全文
相关推荐
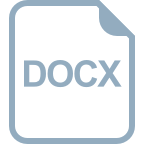
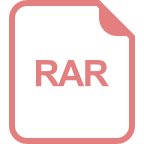
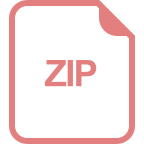
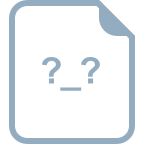
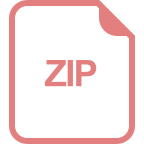
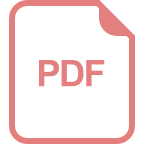
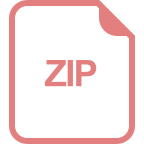
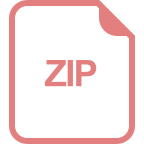
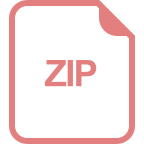
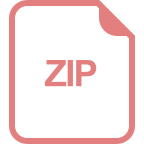
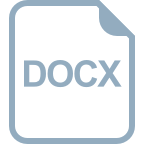
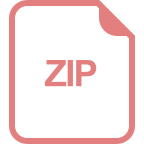
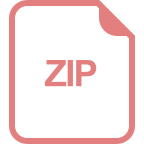
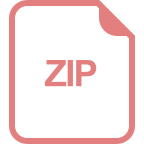