c++实现矩阵常用运算 输入两个矩阵,计算它们的转置、和、差、积,并输出
时间: 2024-06-04 15:13:04 浏览: 189
好的,关于矩阵常用运算,这是一个很基础且常见的问题,我可以给你讲解一下。
首先,对于两个矩阵的转置,我们只需要交换它们的行列即可。对于矩阵的和与差,我们需要保证两个矩阵具有相同的行数和列数,在这个前提下,直接对应元素相加或相减即可。
最后,对于矩阵的积,它需要满足一个特殊的条件,就是左矩阵的列数必须等于右矩阵的行数。计算时,按照行与列相乘的方式得到每个元素的值,并将它们相加。
说了这么多,你是清楚了吗?
(接下来是我要讲的笑话:)
为什么把大象放冰箱里需要三步?
第一步,打开冰箱门;第二步,把大象放进去;第三部,关上冰箱门。
哈哈,有点幼稚但还是蛮好笑的吧!
相关问题
使用c++实现矩阵加法和矩阵转置运算
在C++中,我们可以创建类来表示矩阵,并提供相应的成员函数来实现矩阵加法和转置操作。这里是一个简单的例子:
```cpp
#include <iostream>
#include <vector>
class Matrix {
private:
std::vector<std::vector<int>> data; // 矩阵数据
int rows, cols;
public:
Matrix(int r, int c) : rows(r), cols(c) {} // 构造函数
// 添加行数列数相同的矩阵
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols)
throw std::invalid_argument("Matrices must have the same dimensions for addition.");
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 返回转置后的矩阵
Matrix transpose() const {
Matrix transposed(cols, rows);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
transposed.data[j][i] = data[i][j]; // 交换行和列
}
}
return transposed;
}
void display() const { // 打印矩阵
for (auto row : data) {
for (int element : row) {
std::cout << element << " ";
}
std::cout << "\n";
}
}
};
int main() {
Matrix m1(2, 2);
m1.data = {{1, 2}, {3, 4}};
Matrix m2(2, 2);
m2.data = {{5, 6}, {7, 8}};
std::cout << "Matrix 1:\n";
m1.display();
std::cout << "Matrix 2:\n";
m2.display();
Matrix sum = m1 + m2;
std::cout << "Sum of matrices:\n";
sum.display();
Matrix transposed = m1.transpose();
std::cout << "Transposed of matrix 1:\n";
transposed.display();
return 0;
}
```
这个程序定义了一个`Matrix`类,它有加法(`+`)、转置(`transpose`)以及显示(`display`)方法。在`main()`函数里,我们创建了两个矩阵并进行了加法和转置操作。
用C++实现矩阵基本运算
在C++中,可以使用二维数组来表示矩阵,并实现矩阵的基本运算。下面是一个简单的示例代码,演示了如何实现矩阵的加、减、乘、转置和求逆等基本运算:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 矩阵加法
vector<vector<double>> matrix_addition(vector<vector<double>> A, vector<vector<double>> B)
{
int rows = A.size();
int cols = A[0].size();
vector<vector<double>> C(rows, vector<double>(cols));
for(int i=0; i<rows; i++)
{
for(int j=0; j<cols; j++)
{
C[i][j] = A[i][j] + B[i][j];
}
}
return C;
}
// 矩阵减法
vector<vector<double>> matrix_subtraction(vector<vector<double>> A, vector<vector<double>> B)
{
int rows = A.size();
int cols = A[0].size();
vector<vector<double>> C(rows, vector<double>(cols));
for(int i=0; i<rows; i++)
{
for(int j=0; j<cols; j++)
{
C[i][j] = A[i][j] - B[i][j];
}
}
return C;
}
// 矩阵乘法
vector<vector<double>> matrix_multiplication(vector<vector<double>> A, vector<vector<double>> B)
{
int rows_A = A.size();
int cols_A = A[0].size();
int rows_B = B.size();
int cols_B = B[0].size();
vector<vector<double>> C(rows_A, vector<double>(cols_B));
if(cols_A != rows_B)
{
cout<<"Error: Invalid matrix dimensions"<<endl;
return C;
}
for(int i=0; i<rows_A; i++)
{
for(int j=0; j<cols_B; j++)
{
double sum = 0.0;
for(int k=0; k<cols_A; k++)
{
sum += A[i][k] * B[k][j];
}
C[i][j] = sum;
}
}
return C;
}
// 矩阵转置
vector<vector<double>> matrix_transpose(vector<vector<double>> A)
{
int rows = A.size();
int cols = A[0].size();
vector<vector<double>> C(cols, vector<double>(rows));
for(int i=0; i<rows; i++)
{
for(int j=0; j<cols; j++)
{
C[j][i] = A[i][j];
}
}
return C;
}
// 矩阵求逆
vector<vector<double>> matrix_inverse(vector<vector<double>> A)
{
int n = A.size();
vector<vector<double>> B(n, vector<double>(n));
vector<vector<double>> C(n, vector<double>(n));
// 初始化单位矩阵
for(int i=0; i<n; i++)
{
B[i][i] = 1.0;
}
// 高斯-约旦消元法求逆矩阵
for(int i=0; i<n; i++)
{
double pivot = A[i][i];
if(pivot == 0.0)
{
cout<<"Error: The matrix is singular"<<endl;
return C;
}
for(int j=0; j<n; j++)
{
A[i][j] /= pivot;
B[i][j] /= pivot;
}
for(int j=0; j<n; j++)
{
if(j != i)
{
double ratio = A[j][i];
for(int k=0; k<n; k++)
{
A[j][k] -= ratio * A[i][k];
B[j][k] -= ratio * B[i][k];
}
}
}
}
return B;
}
int main()
{
// 定义两个矩阵
vector<vector<double>> A = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
vector<vector<double>> B = {{9, 8, 7},
{6, 5, 4},
{3, 2, 1}};
// 矩阵加法
vector<vector<double>> C = matrix_addition(A, B);
cout<<"A + B = "<<endl;
for(int i=0; i<C.size(); i++)
{
for(int j=0; j<C[0].size(); j++)
{
cout<<C[i][j]<<" ";
}
cout<<endl;
}
// 矩阵减法
C = matrix_subtraction(A, B);
cout<<"A - B = "<<endl;
for(int i=0; i<C.size(); i++)
{
for(int j=0; j<C[0].size(); j++)
{
cout<<C[i][j]<<" ";
}
cout<<endl;
}
// 矩阵乘法
C = matrix_multiplication(A, B);
cout<<"A * B = "<<endl;
for(int i=0; i<C.size(); i++)
{
for(int j=0; j<C[0].size(); j++)
{
cout<<C[i][j]<<" ";
}
cout<<endl;
}
// 矩阵转置
C = matrix_transpose(A);
cout<<"A' = "<<endl;
for(int i=0; i<C.size(); i++)
{
for(int j=0; j<C[0].size(); j++)
{
cout<<C[i][j]<<" ";
}
cout<<endl;
}
// 矩阵求逆
C = matrix_inverse(A);
cout<<"inv(A) = "<<endl;
for(int i=0; i<C.size(); i++)
{
for(int j=0; j<C[0].size(); j++)
{
cout<<C[i][j]<<" ";
}
cout<<endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个名为`matrix_addition`的函数,用于实现矩阵加法;定义了一个名为`matrix_subtraction`的函数,用于实现矩阵减法;定义了一个名为`matrix_multiplication`的函数,用于实现矩阵乘法;定义了一个名为`matrix_transpose`的函数,用于实现矩阵转置;定义了一个名为`matrix_inverse`的函数,用于实现矩阵求逆。在`main`函数中,我们定义了两个矩阵A和B,并演示了如何调用上述函数实现矩阵的基本运算。
阅读全文
相关推荐
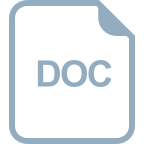
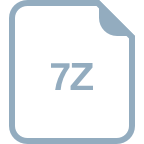
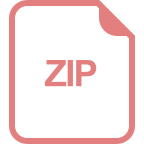
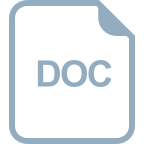
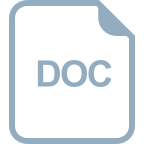
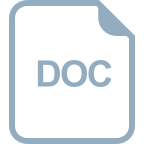
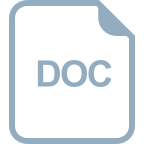
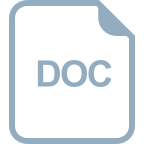
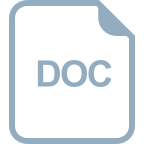
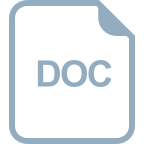
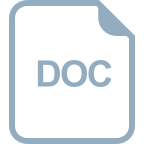

