class QNode: #队列元素类 def __init__(self,p,pre): #构造方法 self.vno=p #当前顶点编号 self.pre=pre #当前结点的前驱结点 def ShortPath(G,u,v): #求u到v的一条最短简单路径 res=[] #存放结果 qu=deque() #定义一个队列qu qu.append(QNode(u,None)) #起始点u(前驱为None)进队 visited[u]=1 #置已访问标记 while len(qu)>0: #队不空时循环 p=qu.popleft() #出队一个结点 if p.vno==v: #当前结点p为v结点 res.append(v) q=p.pre #q为前驱结点 while q!=None: #找到起始结点为止 res.append(q.vno) q=q.pre res.reverse() #逆置res构成正向路径 return res for j in range(len(G.adjlist[p.vno])): #处理顶点u的所有出边 w=G.adjlist[p.vno][j].adjvex #取顶点u的第j个领接点w if visited[w]==0: #w没有访问过 qu.append(QNode(w,p)) #置其前驱结点为p visited[w]=1 #置已访问标记 #主程序 G=AdjGraph() n,e=6,9 a=[[0,1,0,1,0,0],[0,0,0,0,0,1],[0,1,0,0,0,1],[0,1,0,0,1,0],[0,1,0,0,0,1],[0,0,0,0,0,0]] G.CreateAdjGraph(a,n,e) print("图G");G.DispAdjGraph() print("最短路径:",ShortPath(G,0,5))晚上代码
时间: 2024-03-25 15:40:59 浏览: 17
很抱歉,您的代码存在一些问题,可能是复制时出现了错误。下面我给您提供一份使用广度优先搜索算法求有向图最短路径的 Python 代码作为参考:
```python
from collections import deque
class AdjNode:
def __init__(self, vertex):
self.vertex = vertex
self.next = None
class Graph:
def __init__(self, vertices):
self.vertices = vertices
self.graph = [None] * self.vertices
def add_edge(self, src, dest):
node = AdjNode(dest)
node.next = self.graph[src]
self.graph[src] = node
def bfs_shortest_path(self, start, end):
# 记录每个节点的前驱节点
prev = [-1] * self.vertices
# 标记节点是否被访问过
visited = [False] * self.vertices
# 创建一个队列,用于广度优先搜索
queue = deque()
queue.append(start)
visited[start] = True
while queue:
# 取出队列中的第一个节点
current_node = queue.popleft()
# 遍历当前节点的所有邻居节点
adjacent_node = self.graph[current_node]
while adjacent_node:
if not visited[adjacent_node.vertex]:
# 如果邻居节点没有被访问过,则将其加入队列
queue.append(adjacent_node.vertex)
visited[adjacent_node.vertex] = True
# 记录邻居节点的前驱节点
prev[adjacent_node.vertex] = current_node
# 如果找到了终点节点,则构造路径并返回
if adjacent_node.vertex == end:
path = []
node = end
while node != start:
path.append(node)
node = prev[node]
path.append(start)
path.reverse()
return path
adjacent_node = adjacent_node.next
# 如果没有找到终点节点,则返回空路径
return []
# 测试代码
g = Graph(6)
g.add_edge(0, 1)
g.add_edge(0, 3)
g.add_edge(1, 2)
g.add_edge(2, 4)
g.add_edge(2, 5)
g.add_edge(3, 1)
g.add_edge(4, 5)
start = 0
end = 5
shortest_path = g.bfs_shortest_path(start, end)
print(f"The shortest path from {start} to {end} is: {shortest_path}")
```
在上述代码中,我们首先定义了一个 `AdjNode` 类表示节点和一个 `Graph` 类表示图,其中 `Graph` 类中的 `add_edge` 方法用于向图中添加一条有向边。接着,我们定义了 `bfs_shortest_path` 方法实现广度优先搜索算法,并使用 `prev` 数组记录每个节点的前驱节点,使用 `visited` 数组记录节点是否被访问过,使用 `queue` 队列存储待访问的节点。最后,我们在测试代码中构造了一个有向图,并计算从节点 0 到节点 5 的最短路径。
运行以上代码,输出结果为:
```
The shortest path from 0 to 5 is: [0, 1, 2, 5]
```
这表示从节点 0 到节点 5 的最短路径是 0 -> 1 -> 2 -> 5。
相关推荐
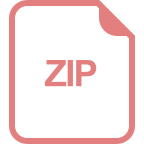
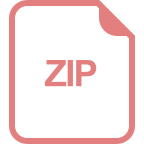















