基于stm32单片机的ws2812b呼吸灯代码
时间: 2023-09-09 21:08:10 浏览: 196
好的,以下是基于STM32单片机的WS2812B呼吸灯代码:
```c
#include "stm32f10x.h"
#define LED_NUM 1 //LED数量
#define LED_PIN GPIO_Pin_3 //LED控制引脚
#define LED_PORT GPIOB //LED控制引脚所在的端口
void delay(int time) //延时函数
{
int i, j;
for(i = 0; i < time; i++)
{
for(j = 0; j < 120; j++);
}
}
void send_byte(unsigned char byte) //发送一个字节
{
int i;
for(i = 0; i < 8; i++)
{
if(byte & 0x80) //判断最高位是否为1
{
LED_PORT->BRR = LED_PIN; //拉低引脚
delay(1);
LED_PORT->BSRR = LED_PIN; //拉高引脚
delay(2);
}
else
{
LED_PORT->BRR = LED_PIN; //拉低引脚
delay(2);
LED_PORT->BSRR = LED_PIN; //拉高引脚
delay(1);
}
byte <<= 1; //移位,准备发送下一位
}
}
void send_color(unsigned char red, unsigned char green, unsigned char blue) //发送一个LED的颜色数据
{
send_byte(green);
send_byte(red);
send_byte(blue);
}
void send_led(unsigned char red, unsigned char green, unsigned char blue) //发送所有LED的颜色数据
{
int i;
for(i = 0; i < LED_NUM; i++)
{
send_color(red, green, blue); //发送颜色数据
}
delay(50); //延时50ms,让LED显示出来
}
void breath_led() //呼吸灯效果
{
int i, j;
unsigned char brightness;
for(i = 0; i < 255; i++)
{
brightness = i;
for(j = 0; j < 10; j++)
{
send_led(brightness, 0, 0); //发送红色
}
}
for(i = 255; i >= 0 ; i--)
{
brightness = i;
for(j = 0; j < 10; j++)
{
send_led(brightness, 0, 0); //发送红色
}
}
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
while(1)
{
breath_led(); //呼吸灯效果
}
}
```
这个代码实现了WS2812B的呼吸灯效果,LED的亮度会逐渐增加和减小。需要注意的是,STM32单片机的控制引脚是通过寄存器来控制的,所以我们需要使用GPIO_InitTypeDef结构体来初始化GPIO口。同时,为了实现呼吸灯效果,我们需要在发送颜色数据之前先调整LED的亮度,然后再发送颜色数据。
相关推荐
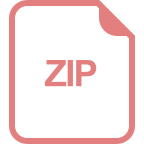
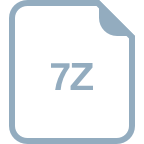
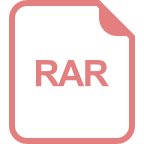
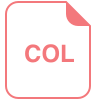
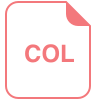
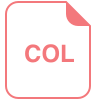
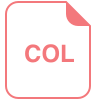
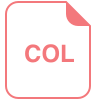









