linux c 获取串口的数据然后解析代码
时间: 2023-11-04 07:04:19 浏览: 262
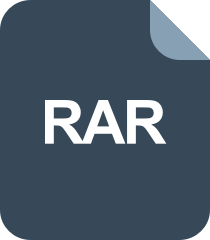
linux下串口编程及非标准波特率的实现代码
以下是一个简单的Linux C语言示例,演示如何获取串口数据并进行解析。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
char *portName = "/dev/ttyUSB0"; // 串口名称
struct termios options;
// 打开串口
fd = open(portName, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
printf("Failed to open port\n");
return 1;
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B115200); // 输入波特率
cfsetospeed(&options, B115200); // 输出波特率
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8; // 数据位数
options.c_cflag &= ~PARENB; // 校验位
options.c_cflag &= ~CSTOPB; // 停止位
tcsetattr(fd, TCSANOW, &options);
// 读取数据
char buffer[1024]; // 接收缓冲区
while (1) {
int count = read(fd, buffer, sizeof(buffer));
if (count > 0) {
// 解析数据
for (int i = 0; i < count; i++) {
printf("%02X ", buffer[i]); // 打印接收到的数据,以16进制格式输出
}
printf("\n");
}
usleep(100000); // 等待一段时间再继续读取数据
}
// 关闭串口
close(fd);
return 0;
}
```
以上代码演示了如何打开串口、配置串口参数、读取数据以及解析数据。具体的解析方法需要根据你的数据格式而定。
阅读全文
相关推荐
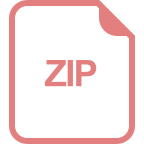
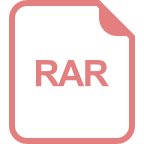















