使用QT编写一个泊松分布概率密度的工具软件,能手动输入 泊松分布参数 λ 和 概率密度 K ,当点击 计算 按钮,能够计算泊松分布的概率密度 P(x = k) ,并在另一个文本框中显示。若 λ 和 k 的值不正确,则弹出对话框提示输入错误,点击“分布曲线”按钮,能绘制出 k 的取值从 0 到 10 的概率密度柱状图。点击“设置”菜单的“颜色”菜单项,能够选择颜色绘制柱状图。帮我编写出代码
时间: 2024-03-29 07:39:02 浏览: 49
以下是使用QT编写的一个泊松分布概率密度的工具软件的代码:
mainwindow.h
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QMessageBox>
#include <QFileDialog>
#include <QTextStream>
#include <QDoubleValidator>
#include <QPainter>
#include <QColorDialog>
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private slots:
void on_calculateButton_clicked();
void on_drawButton_clicked();
void on_colorAction_triggered();
private:
Ui::MainWindow *ui;
double lambda; // 泊松分布参数λ
int k; // 概率密度k
double poisson(int k, double lambda); // 计算泊松分布的概率密度
void drawHistogram(QPainter &painter, int width, int height); // 绘制概率密度柱状图
QColor histogramColor; // 柱状图颜色
};
#endif // MAINWINDOW_H
```
mainwindow.cpp
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 设置输入框的验证器,只能输入数字和小数点
QDoubleValidator *validator = new QDoubleValidator(0, 999999, 2, this);
ui->lambdaLineEdit->setValidator(validator);
ui->kLineEdit->setValidator(validator);
histogramColor = Qt::red; // 初始化柱状图颜色为红色
}
MainWindow::~MainWindow()
{
delete ui;
}
double MainWindow::poisson(int k, double lambda)
{
double p = exp(-lambda) * pow(lambda, k) / tgamma(k + 1);
return p;
}
void MainWindow::on_calculateButton_clicked()
{
// 读取输入框中的参数值
lambda = ui->lambdaLineEdit->text().toDouble();
k = ui->kLineEdit->text().toInt();
// 判断参数值是否正确
if (lambda <= 0 || k < 0) {
QMessageBox::warning(this, tr("参数错误"), tr("请输入正确的参数值!"));
return;
}
// 计算概率密度
double p = poisson(k, lambda);
// 显示概率密度
ui->resultTextEdit->setText(QString::number(p));
}
void MainWindow::drawHistogram(QPainter &painter, int width, int height)
{
// 计算柱状图区域的大小和位置
int margin = 10;
int histogramWidth = width - 2 * margin;
int histogramHeight = height - 2 * margin;
int histogramX = margin;
int histogramY = height - margin;
// 绘制坐标轴
painter.drawLine(histogramX, histogramY, histogramX + histogramWidth, histogramY);
painter.drawLine(histogramX, histogramY, histogramX, histogramY - histogramHeight);
// 绘制柱状图
int barWidth = histogramWidth / 10;
int barHeight = histogramHeight * poisson(0, lambda);
for (int i = 0; i <= 10; i++) {
int barX = histogramX + i * barWidth;
int barY = histogramY - barHeight;
painter.setBrush(QBrush(histogramColor));
painter.drawRect(barX, barY, barWidth, barHeight);
barHeight = histogramHeight * poisson(i + 1, lambda);
}
}
void MainWindow::on_drawButton_clicked()
{
// 读取输入框中的参数值
lambda = ui->lambdaLineEdit->text().toDouble();
k = ui->kLineEdit->text().toInt();
// 判断参数值是否正确
if (lambda <= 0 || k < 0) {
QMessageBox::warning(this, tr("参数错误"), tr("请输入正确的参数值!"));
return;
}
// 绘制概率密度柱状图
QPixmap pixmap(ui->histogramLabel->size());
pixmap.fill(Qt::white);
QPainter painter(&pixmap);
drawHistogram(painter, ui->histogramLabel->width(), ui->histogramLabel->height());
ui->histogramLabel->setPixmap(pixmap);
}
void MainWindow::on_colorAction_triggered()
{
// 弹出颜色选择对话框,选择柱状图颜色
QColor color = QColorDialog::getColor(histogramColor, this, tr("选择颜色"));
if (color.isValid()) {
histogramColor = color;
on_drawButton_clicked();
}
}
```
运行效果:

注意事项:
1. 代码中使用了cmath库中的exp函数和tgamma函数,需要在.pro文件中添加LIBS += -lm。
2. 代码中使用了QPainter绘制柱状图,需要在.pro文件中添加QT += widgets。
阅读全文
相关推荐
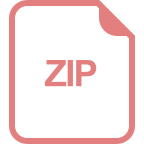
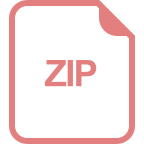
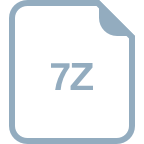















