完成时间片轮转算法程序的基本步骤: a) 每个PCB模块的基本信息:进程名称,需要运行的时间,已用CPU时间,进程状态,时间片。 b) 程序的基本模块: 1> 进程的创建 由create2()函数完成。采用链表的方法创建五个进程,进程名和进程所需运行的时间,并初始化其状态为w(等待),每个时间片的运行时间为2,且把就绪队列的第一个进程放入CPU运行,使就绪队列的头指针指向第二个进程。 2> 轮转法插入函数,当一个时间片的时间已到,而进程又未完成就需把进程插入到就绪队列的尾部,该模块由insert 函数完成。 3> 时间片轮转法的实现 由roundrun函数完成。程序记下进程的运行时间,并计算剩下需运行的时间。如果剩下需运行的时间变为0时就把它插到完成队列,设其运行状态为F(完成)。如果时间片的时间到了而进程还未完成,就把它插到就绪队列的队尾,同时把就绪队列的第一个进程投入运行。 4> 输出模块。包括标题的输出,进程的输出,运行过程的输出
时间: 2024-02-12 19:05:52 浏览: 116
下面是一个简单的 C 语言实现时间片轮转算法程序的代码,按照上述基本步骤实现。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TIME_QUANTUM 2
typedef struct PCB {
char name[10];
int need_time;
int used_time;
char status;
struct PCB *next;
} PCB;
PCB *create(char *name, int need_time) {
PCB *process = (PCB *)malloc(sizeof(PCB));
strcpy(process->name, name);
process->need_time = need_time;
process->used_time = 0;
process->status = 'W'; // 等待状态
process->next = NULL;
return process;
}
void insert(PCB **head, PCB *process) {
if (*head == NULL) {
*head = process;
return;
}
PCB *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = process;
}
void roundrun(PCB **head, PCB **finished, int *time) {
PCB *running = *head;
*head = running->next;
running->next = NULL;
printf("Running process: %s\n", running->name);
int run_time = running->need_time - running->used_time;
if (run_time > TIME_QUANTUM) {
running->used_time += TIME_QUANTUM;
*time += TIME_QUANTUM;
run_time -= TIME_QUANTUM;
printf("Process %s runs %d seconds.\n", running->name, TIME_QUANTUM);
printf("Process %s needs %d seconds to finish.\n", running->name, run_time);
insert(head, running);
} else {
running->used_time += run_time;
*time += run_time;
running->status = 'F'; // 完成状态
printf("Process %s runs %d seconds and finished.\n", running->name, run_time);
insert(finished, running);
}
}
void print_processes(PCB *head) {
printf("Processes:\n");
printf("Name\tNeed Time\tUsed Time\tStatus\n");
PCB *p = head;
while (p != NULL) {
printf("%s\t%d\t\t%d\t\t%c\n", p->name, p->need_time, p->used_time, p->status);
p = p->next;
}
}
void print_finished(PCB *head) {
printf("Finished Processes:\n");
printf("Name\tNeed Time\tUsed Time\n");
PCB *p = head;
while (p != NULL) {
printf("%s\t%d\t\t%d\n", p->name, p->need_time, p->used_time);
p = p->next;
}
}
int main() {
PCB *head = NULL;
PCB *finished = NULL;
PCB *p1 = create("P1", 5);
PCB *p2 = create("P2", 2);
PCB *p3 = create("P3", 3);
PCB *p4 = create("P4", 4);
PCB *p5 = create("P5", 1);
insert(&head, p1);
insert(&head, p2);
insert(&head, p3);
insert(&head, p4);
insert(&head, p5);
int time = 0;
while (head != NULL) {
roundrun(&head, &finished, &time);
}
printf("Total Time: %d seconds.\n", time);
print_processes(finished);
return 0;
}
```
该程序创建了五个进程,采用链表的方式,每个时间片的运行时间为 2 秒。程序的输出包括运行过程、进程信息和完成的进程信息。
阅读全文
相关推荐
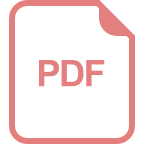
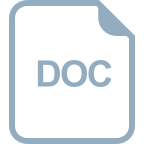
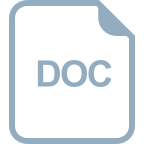
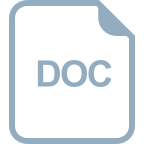
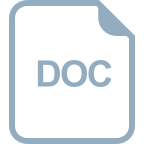
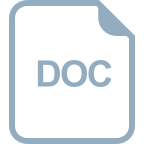
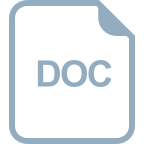
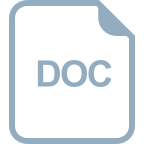
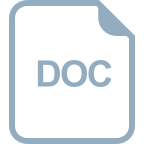
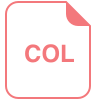
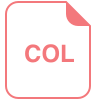
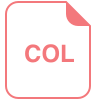
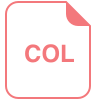
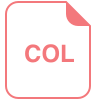
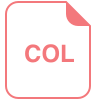
