输入一组包含不同类型括号的运算表达式,括号可以有()、{}、[],注意:请使用英文括号,括号在表达式配对中能够正确匹配,是否会存在不合理的括号嵌套,是否会存在无法配对的括号,如果判断成果,输出表达式判断为True,如果失败输出False。
时间: 2024-12-23 13:27:02 浏览: 3
Given an expression containing various types of parentheses, such as ( ), { }, and [], the task is to validate whether these parentheses are correctly matched and form a valid balanced sequence. A balanced sequence means that every opening parenthesis has a corresponding closing one, and no unmatched or improperly nested parentheses exist.
To accomplish this, you can use a stack data structure. Here's a basic approach:
1. Iterate through each character in the expression.
2. If the character is an opening parenthesis (i.e., '(', '{', or '['), push it onto the stack.
3. If the character is a closing parenthesis, check if the stack is not empty. If it is, pop the top element from the stack and compare it with the current closing parenthesis. If they match (e.g., ')' matches '(', '{' matches '}', etc.), continue; otherwise, the expression is invalid and return False.
4. If the stack is empty after iterating through all characters, but there are still some closing parentheses left, it indicates unmatched opening parentheses and returns False.
5. If the stack is empty at the end, the expression is considered balanced and returns True.
Here's a simple Python function demonstrating this logic:
```python
def is_valid_parentheses(expression):
brackets_map = {
")": "(",
"}": "{",
"]": "["
}
stack = []
for char in expression:
if char in brackets_map:
if not stack or stack[-1] != brackets_map[char]:
return False
stack.pop()
else:
stack.append(char)
return not stack
# Example usage:
expression_1 = "(){}[]" # Balanced, so True
expression_2 = "({[})" # Unbalanced, so False
expression_3 = "[(]" # Unmatched opening, so False
print(is_valid_parentheses(expression_1)) # Output: True
print(is_valid_parentheses(expression_2)) # Output: False
print(is_valid_parentheses(expression_3)) # Output: False
```
阅读全文
相关推荐
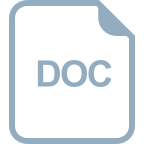
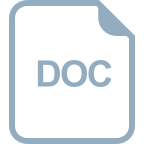
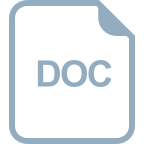




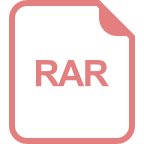
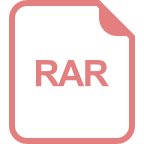









