python requests json
时间: 2023-04-24 16:03:10 浏览: 135
Python中的requests库可以用来发送HTTP请求,其中包括发送JSON数据。使用requests库发送JSON数据时,需要将数据转换为JSON格式,并设置请求头中的Content-Type为application/json。具体实现可以参考以下代码:
```python
import requests
import json
url = 'http://example.com/api'
data = {'name': 'John', 'age': 30}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(data), headers=headers)
```
其中,url为请求的地址,data为要发送的JSON数据,headers为请求头。使用requests.post()方法发送POST请求,并将JSON数据转换为字符串后作为参数传入。最后,可以通过response对象获取服务器返回的响应。
相关问题
python requests json参数
Python中的requests库可以使用JSON参数来发送HTTP请求。JSON参数是一种轻量级的数据交换格式,可以在不同的编程语言和平台之间进行数据交换。在requests库中,可以使用json参数来发送POST请求,将JSON数据作为请求体发送给服务器。例如:
```python
import requests
url = 'http://example.com/api'
data = {'name': 'John', 'age': 30}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, json=data, headers=headers)
print(response.json())
```
在上面的代码中,我们使用requests.post()方法发送了一个POST请求,将JSON数据作为请求体发送给服务器。json参数接受一个Python字典作为输入,将其转换为JSON格式并发送给服务器。我们还可以通过headers参数设置请求头,指定请求体的数据类型为JSON。最后,我们使用response.json()方法获取服务器返回的JSON数据。
python requests json 和params
在使用Python的requests库发送HTTP请求时,可以使用`json`参数和`params`参数来传递数据。
1. `json`参数:可以将Python对象转换为JSON格式,并将其作为请求体中的数据进行发送。使用`json`参数发送POST请求示例代码如下:
```python
import requests
url = 'https://example.com/api'
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=payload)
```
2. `params`参数:可以将参数添加到URL中,用于GET请求或者作为查询字符串的一部分。使用`params`参数发送GET请求示例代码如下:
```python
import requests
url = 'https://example.com/api'
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get(url, params=params)
```
阅读全文
相关推荐
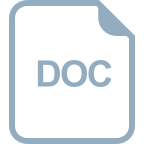
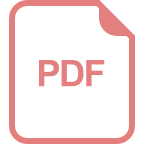



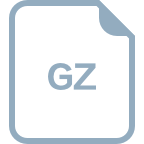
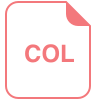







