检查代码import random total=1000000 in_count=0 for i in range(total): x=random.uniform(0,1) y=random.uniform(0,1) dis=(x**2+y**2)**0.5 if dis<=1: in_count+=1 print('pi is:',4*in_count/total)
时间: 2024-04-09 15:31:57 浏览: 21
这段代码是用蒙特卡洛方法来估算圆周(pi)的值。它通过在正方形内随机生成点,并判断些点是否落在单位圆内来估算。代码中的变量`total表示生成的点的总数,`in_count`表示落在单位圆内的点的数量。
循环中,每次生成两0到1之间的机数,分别点的x和y坐。然后计算点到原点的距离`dis`如果距离小于等于1,则将in_count`加1。
最后,计算`4*in_count/total`来估算圆周率(pi)的值,并将结果打印出来。
请问有什么问题我可以帮您解答吗?
相关问题
生成 遗传算法优化粒子群算法代码
以下是一个简单的遗传算法优化粒子群算法的代码示例(仅供参考):
```python
import random
# 粒子群算法类
class PSO:
def __init__(self, particle_count, dimensions, fitness_func, max_iterations=100, c1=2.0, c2=2.0, w=1.0):
self.particle_count = particle_count
self.dimensions = dimensions
self.fitness_func = fitness_func
self.max_iterations = max_iterations
self.c1 = c1
self.c2 = c2
self.w = w
self.global_best_position = [0.0] * dimensions
self.global_best_fitness = float("inf")
self.particles = []
# 初始化粒子群
for i in range(particle_count):
particle = Particle(dimensions)
self.particles.append(particle)
# 运行粒子群算法
def run(self):
for i in range(self.max_iterations):
for particle in self.particles:
fitness = self.fitness_func(particle.position)
if fitness < particle.best_fitness:
particle.best_fitness = fitness
particle.best_position = particle.position
if fitness < self.global_best_fitness:
self.global_best_fitness = fitness
self.global_best_position = particle.position
for particle in self.particles:
particle.update_velocity(self.global_best_position, self.c1, self.c2, self.w)
particle.update_position()
# 粒子类
class Particle:
def __init__(self, dimensions):
self.position = [random.uniform(-5.0, 5.0) for i in range(dimensions)]
self.velocity = [0.0] * dimensions
self.best_position = self.position
self.best_fitness = float("inf")
# 更新速度
def update_velocity(self, global_best_position, c1, c2, w):
for i in range(len(self.position)):
r1 = random.random()
r2 = random.random()
cognitive_velocity = c1 * r1 * (self.best_position[i] - self.position[i])
social_velocity = c2 * r2 * (global_best_position[i] - self.position[i])
self.velocity[i] = w * self.velocity[i] + cognitive_velocity + social_velocity
# 更新位置
def update_position(self):
for i in range(len(self.position)):
self.position[i] += self.velocity[i]
# 适应度函数
def fitness_func(position):
# TODO: 计算适应度值
return fitness_value
# 遗传算法类
class GA:
def __init__(self, population_size, dimensions, fitness_func, max_generations=100, crossover_rate=0.8, mutation_rate=0.1):
self.population_size = population_size
self.dimensions = dimensions
self.fitness_func = fitness_func
self.max_generations = max_generations
self.crossover_rate = crossover_rate
self.mutation_rate = mutation_rate
self.population = []
self.best_individual = None
self.best_fitness = float("inf")
# 初始化种群
for i in range(population_size):
individual = Individual(dimensions)
self.population.append(individual)
# 运行遗传算法
def run(self):
for generation in range(self.max_generations):
# 计算适应度值
for individual in self.population:
fitness = self.fitness_func(individual.genes)
individual.fitness = fitness
if fitness < self.best_fitness:
self.best_fitness = fitness
self.best_individual = individual
# 选择
new_population = []
total_fitness = sum([individual.fitness for individual in self.population])
for i in range(self.population_size):
roulette_wheel_position = random.uniform(0, total_fitness)
spin_wheel = 0
for individual in self.population:
spin_wheel += individual.fitness
if spin_wheel >= roulette_wheel_position:
new_population.append(individual)
break
# 交叉
for i in range(0, self.population_size - 1, 2):
if random.random() < self.crossover_rate:
crossover_point = random.randint(1, self.dimensions - 2)
new_population[i].genes[crossover_point:], new_population[i+1].genes[crossover_point:] = \
new_population[i+1].genes[crossover_point:], new_population[i].genes[crossover_point:]
# 变异
for individual in new_population:
for i in range(self.dimensions):
if random.random() < self.mutation_rate:
individual.genes[i] = random.uniform(-5.0, 5.0)
self.population = new_population
# 个体类
class Individual:
def __init__(self, dimensions):
self.genes = [random.uniform(-5.0, 5.0) for i in range(dimensions)]
self.fitness = 0
# 运行示例
def main():
pso = PSO(particle_count=50, dimensions=10, fitness_func=fitness_func)
pso.run()
ga = GA(population_size=50, dimensions=10, fitness_func=fitness_func)
ga.run()
if __name__ == "__main__":
main()
```
在上述代码中,PSO 和 GA 分别实现了粒子群算法和遗传算法,并且均包含了相应的粒子/个体类。在主函数中,我们可以分别实例化 PSO 和 GA,并通过调用其 run() 方法来运行算法。请注意,上述代码仅为示例,实际应用中需要根据具体问题进行适当修改。
python解决车间调度问题代码
根据提供的引用,车间调度问题是一个经典的NP-hard问题,需要使用复杂的算法进行求解。以下是一个使用遗传算法求解车间调度问题的Python代码示例:
```python
import random
# 定义遗传算法的参数
POPULATION_SIZE = 50 # 种群大小
CROSSOVER_RATE = 0.9 # 交叉概率
MUTATION_RATE = 0.1 # 变异概率
GENERATION_COUNT = 100 # 迭代次数
# 定义车间调度问题的参数
MACHINE_COUNT = 3 # 机器数量
JOB_COUNT = 6 # 工件数量
OPERATION_COUNT = 2 # 工序数量
JOBS = [i for i in range(JOB_COUNT)]
MACHINES = [i for i in range(MACHINE_COUNT)]
OPERATIONS = [i for i in range(OPERATION_COUNT)]
# 定义工件的加工时间
PROCESSING_TIMES = [
[[3, 2, 1], [2, 1, 2]],
[[2, 3, 1], [1, 2, 1]],
[[1, 2, 3], [2, 3, 1]],
[[3, 1, 2], [1, 3, 2]],
[[2, 1, 3], [3, 2, 1]],
[[1, 3, 2], [2, 1, 3]]
]
# 定义种群类
class Population:
def __init__(self, size):
self.size = size
self.individuals = []
for i in range(size):
individual = Individual()
self.individuals.append(individual)
def get_fittest(self):
fittest = self.individuals[0]
for i in range(1, self.size):
if self.individuals[i].fitness > fittest.fitness:
fittest = self.individuals[i]
return fittest
# 定义个体类
class Individual:
def __init__(self):
self.genes = []
for i in range(JOB_COUNT):
gene = Gene()
self.genes.append(gene)
self.fitness = self.calculate_fitness()
def calculate_fitness(self):
makespan = 0
machine_time = [0] * MACHINE_COUNT
for i in range(JOB_COUNT * OPERATION_COUNT):
job_index = self.genes[i].job_index
machine_index = self.genes[i].machine_index
processing_time = PROCESSING_TIMES[job_index][self.genes[i].operation_index][machine_index]
start_time = max(machine_time[machine_index], makespan)
end_time = start_time + processing_time
machine_time[machine_index] = end_time
makespan = max(makespan, end_time)
return 1 / makespan
def crossover(self, other):
child1 = Individual()
child2 = Individual()
for i in range(JOB_COUNT):
if random.random() < CROSSOVER_RATE:
child1.genes[i] = self.genes[i]
child2.genes[i] = other.genes[i]
else:
child1.genes[i] = other.genes[i]
child2.genes[i] = self.genes[i]
return child1, child2
def mutate(self):
for i in range(JOB_COUNT):
if random.random() < MUTATION_RATE:
self.genes[i] = Gene()
# 定义基因类
class Gene:
def __init__(self):
self.job_index = random.choice(JOBS)
self.machine_index = random.choice(MACHINES)
self.operation_index = random.choice(OPERATIONS)
# 定义遗传算法函数
def genetic_algorithm():
population = Population(POPULATION_SIZE)
for i in range(GENERATION_COUNT):
new_population = Population(POPULATION_SIZE)
for j in range(POPULATION_SIZE // 2):
parent1 = roulette_wheel_selection(population)
parent2 = roulette_wheel_selection(population)
child1, child2 = parent1.crossover(parent2)
child1.mutate()
child2.mutate()
new_population.individuals[j * 2] = child1
new_population.individuals[j * 2 + 1] = child2
population = new_population
return population.get_fittest()
# 定义轮盘赌选择函数
def roulette_wheel_selection(population):
total_fitness = sum([individual.fitness for individual in population.individuals])
random_value = random.uniform(0, total_fitness)
current_sum = 0
for individual in population.individuals:
current_sum += individual.fitness
if current_sum > random_value:
return individual
# 运行遗传算法并输出结果
best_individual = genetic_algorithm()
print("Best makespan: ", 1 / best_individual.fitness)
```
以上代码使用遗传算法求解车间调度问题,其中定义了种群、个体、基因等类,并实现了交叉、变异、选择等操作。在遗传算法函数中,首先初始化种群,然后进行迭代,每次迭代都进行交叉、变异、选择等操作,最终返回最优个体。
相关推荐
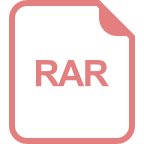












