% 加密明文plaintext = 'jnnjvbdsjbikaBHGXnjgFDT';ciphertext = replace(plaintext, alphabet, replace_table);disp(['密文:', ciphertext]);% 保存密文到文件fid = fopen('ok.txt', 'wt');fprintf(fid, '%s', ciphertext);fclose(fid);如何在上面代码中用strrep函数代替replace函数
时间: 2023-12-22 13:02:53 浏览: 27
可以将 `replace` 函数替换为 `strrep` 函数,如下所示:
```
% 定义明文、字母表和替换表
plaintext = 'jnnjvbdsjbikaBHGXnjgFDT';
alphabet = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
replace_table = 'defghijklmnopqrstuvwxyzabcDEFGHIJKLMNOPQRSTUVWXYZABC';
% 加密明文
ciphertext = strrep(plaintext, alphabet, replace_table);
disp(['密文:', ciphertext]);
% 保存密文到文件
fid = fopen('ok.txt', 'wt');
fprintf(fid, '%s', ciphertext);
fclose(fid);
```
`strrep` 函数的使用方式与 `replace` 函数类似,都是将字符串中的某些子串替换为另外一个子串,只是函数名和参数顺序略有不同。在这个例子中,我们直接将 `replace` 函数替换为 `strrep` 函数,并将参数的顺序稍作调整,就可以完成替换操作了。
相关问题
如何matlab实现,简单替换密码的替换表。利用简单替换密码算法对明文‘jnnjvbdsjbikaBHGXnjgFDT’进行加密,形成密文ok.txt,然后解密并形成解密文件
实现简单替换密码的替换表可以使用Matlab中的字符串处理函数实现,例如可以使用char函数将数字和字母转换为ASCII码,然后进行简单的加减运算,得到替换后的字符。
以下是一个示例代码,用于生成简单替换密码的替换表:
```matlab
% 生成简单替换密码的替换表
alphabet = 'abcdefghijklmnopqrstuvwxyz';
num = '0123456789';
replace_table = [num alphabet upper(alphabet)];
for i = 1:length(replace_table)
replace_table(i) = char(replace_table(i) + 10);
end
disp(['原始字符集:', alphabet, num, upper(alphabet)]);
disp(['替换字符集:', replace_table]);
```
对于明文'jnnjvbdsjbikaBHGXnjgFDT'进行加密,可以使用以下代码:
```matlab
% 加密明文
plaintext = 'jnnjvbdsjbikaBHGXnjgFDT';
ciphertext = replace(plaintext, alphabet, replace_table);
disp(['密文:', ciphertext]);
% 保存密文到文件
fid = fopen('ok.txt', 'wt');
fprintf(fid, '%s', ciphertext);
fclose(fid);
```
其中,replace是Matlab中的字符串替换函数,用于将明文中的每一个字符按照替换表进行替换。
对于密文进行解密,可以使用以下代码:
```matlab
% 读取密文文件
fid = fopen('ok.txt', 'rt');
ciphertext = fscanf(fid, '%s');
fclose(fid);
% 解密密文
plaintext = replace(ciphertext, replace_table, alphabet);
disp(['解密明文:', plaintext]);
% 保存解密明文到文件
fid = fopen('decrypt.txt', 'wt');
fprintf(fid, '%s', plaintext);
fclose(fid);
```
其中,replace函数的第二个参数和第三个参数交换位置,用于将密文中的每一个字符按照替换表进行还原。最后将解密明文保存到文件'decrypt.txt'中。
单表代换: 实验目的:通过实验熟练掌握移位密码原理,编程实现加密算法,提高程序设计能力,掌握穷举破译的方法。 实验要求: 用python实现(1)输入任意的一段明文,对其加密并输出密文; (2)输入一段密文,利用穷举法进行唯密文攻击,输出密钥。
以下是单表代换加密算法的Python实现,包括明文加密和密文解密:
```python
import string
import random
def generate_key():
# 生成随机密钥表
alphabet = list(string.ascii_lowercase)
random.shuffle(alphabet)
return ''.join(alphabet)
def encrypt(plaintext, key):
# 加密明文
ciphertext = ''
for char in plaintext:
if char.isalpha():
index = ord(char.lower()) - ord('a')
ciphertext += key[index]
else:
ciphertext += char
return ciphertext
def decrypt(ciphertext, key):
# 解密密文
plaintext = ''
for char in ciphertext:
if char.isalpha():
index = key.index(char.lower())
plaintext += chr(index + ord('a'))
else:
plaintext += char
return plaintext
# 测试加密
plaintext = 'hello world'
key = generate_key()
ciphertext = encrypt(plaintext, key)
print('明文:', plaintext)
print('密钥:', key)
print('密文:', ciphertext)
# 测试解密
plaintext = decrypt(ciphertext, key)
print('解密后的明文:', plaintext)
```
以下是唯密文攻击的Python实现:
```python
def brute_force(ciphertext):
# 穷举密钥表
for i in range(26):
key = ''
for j in range(26):
index = (j + i) % 26
key += chr(index + ord('a'))
plaintext = decrypt(ciphertext, key)
print('尝试密钥:', key)
print('解密后的明文:', plaintext)
# 测试唯密文攻击
ciphertext = 'uryyb jbeyq'
brute_force(ciphertext)
```
在唯密文攻击中,我们通过穷举所有可能的密钥表,将密文解密为明文。上面的代码输出了26个尝试的密钥表和对应的明文,其中只有一个是正确的密钥表。
相关推荐
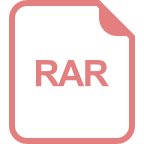
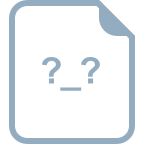












