基于图像的苹果检测代码
时间: 2023-06-14 14:04:23 浏览: 49
以下是基于深度学习模型的苹果检测代码,使用的是 TensorFlow 和 OpenCV 库:
首先,需要安装 TensorFlow 和 OpenCV 库,可以使用以下命令:
```
pip install tensorflow opencv-python
```
然后,下载预训练的模型文件,这里使用的是 SSD 模型,可以从 TensorFlow 官方网站下载:http://download.tensorflow.org/models/object_detection/ssd_mobilenet_v1_coco_2018_01_28.tar.gz
解压之后,将其中的 frozen_inference_graph.pb 文件复制到当前目录下。
接下来,使用以下代码进行苹果检测:
```python
import cv2
import numpy as np
import tensorflow as tf
# 加载模型
model_path = 'frozen_inference_graph.pb'
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(model_path, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
# 定义类别标签
category_index = {1: {'id': 1, 'name': 'apple'}}
# 检测函数
def detect_apple(image):
with detection_graph.as_default():
with tf.Session(graph=detection_graph) as sess:
# 输入张量
image_tensor = detection_graph.get_tensor_by_name('image_tensor:0')
# 检测框张量
detection_boxes = detection_graph.get_tensor_by_name('detection_boxes:0')
# 分数张量
detection_scores = detection_graph.get_tensor_by_name('detection_scores:0')
# 类别标签张量
detection_classes = detection_graph.get_tensor_by_name('detection_classes:0')
# 执行检测
(boxes, scores, classes) = sess.run(
[detection_boxes, detection_scores, detection_classes],
feed_dict={image_tensor: image})
# 获取检测框
boxes = np.squeeze(boxes)
# 获取分数
scores = np.squeeze(scores)
# 获取类别标签
classes = np.squeeze(classes).astype(np.int32)
# 仅保留分数大于阈值的检测结果
threshold = 0.5
idx = np.where(scores > threshold)[0]
boxes = boxes[idx]
scores = scores[idx]
classes = classes[idx]
# 绘制检测结果
height, width, _ = image.shape
for i in range(len(boxes)):
ymin, xmin, ymax, xmax = boxes[i]
left = int(xmin * width)
top = int(ymin * height)
right = int(xmax * width)
bottom = int(ymax * height)
cv2.rectangle(image, (left, top), (right, bottom), (0, 255, 0), 2)
class_name = category_index[classes[i]]['name']
score = scores[i]
label = '{}: {:.2f}'.format(class_name, score)
cv2.putText(image, label, (left, top - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 1, cv2.LINE_AA)
return image
# 读取图像并进行检测
image = cv2.imread('apple.jpg')
image = detect_apple(image)
cv2.imshow('image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,这里假设图像中只有一个苹果,如果图像中有多个苹果,需要对每个苹果进行循环检测。
相关推荐
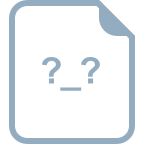














