ValueError: Index out of range using input dim 4; input has only 4 dims for '{{node network/strided_slice}} = StridedSlice[Index=DT_INT32, T=DT_FLOAT, begin_mask=23, ellipsis_mask=0, end_mask=23, new_axis_mask=0, shrink_axis_mask=8](network/spatial_conv/tf_op_layer_concat_1/concat_1, network/strided_slice/stack, network/strided_slice/stack_1, network/strided_slice/stack_2)' with input shapes: [1,20,20,60], [5], [5], [5] and with computed input tensors: input[3] = <1 1 1 1 1>.
时间: 2024-03-29 14:39:13 浏览: 362
这是一个 ValueError 错误,它表示您正在尝试使用的索引超出了张量的范围。根据错误消息,您正在尝试使用具有四个维度的张量进行切片,但是索引张量中的某些值超出了张量可用的范围。您需要检查索引张量的值是否正确,并确保它们不超出张量的范围。您还可以检查您的代码,以查看是否有任何错误,例如使用错误的张量形状或错误的切片索引。
相关问题
def tr_encoder(self, encoder_input, encoder_mask, hidden_size=256, head_num=4, hidden_layer_num=12, intermediate_size=2048): if hidden_size % head_num != 0: raise ValueError(f'hidden_size:{hidden_size} num_attention_heads:{head_num}') head_dim = int(hidden_size / head_num) all_layer_outputs = [] for layer_idx in range(hidden_layer_num): # encoder-self-attention residual = encoder_input encoder_output = layers.LayerNormalization(epsilon=1e-5)(encoder_input) query, key, value = self.compute_qkv(name=f'encoder_qkv_{layer_idx}', query=encoder_output, key=encoder_output, value=encoder_output, head_num=head_num, head_dim=head_dim) scores = self.compute_score(query=query, key=key, head_dim=head_dim) encoder_attention_mask = tf.expand_dims(tf.expand_dims(encoder_mask, 1), 1) encoder_output = self.compute_attention_result(value=value, scores=scores, mask=encoder_attention_mask, head_num=head_num, head_dim=head_dim) encoder_output = layers.Dense(units=hidden_size, kernel_initializer='he_normal')(encoder_output) encoder_output = layers.Dropout(0.1)(encoder_output) encoder_output = layers.Add()([residual, encoder_output])
这是一个 transformer 编码器的实现,用于对输入进行编码。它包含多个 transformer 编码层,每个层都包含 self-attention 和前向网络两个子层。具体来说,对于每个层,它的输入是 encoder_input 和 encoder_mask,其中 encoder_mask 是一个掩码矩阵,用于指示哪些位置是有效的。在 self-attention 子层中,它首先对输入进行 layer normalization,然后计算 query、key 和 value,再计算 attention 分数,最后通过 attention 分数、value 和掩码计算出 attention 输出。在前向网络子层中,它将 attention 输出作为输入,并依次进行全连接、dropout 和残差连接操作。最后,它返回所有层的输出。
ValueError: Dimensions must be equal, but are 2 and 3 for '{{node mean_squared_error/SquaredDifference}} = SquaredDifference[T=DT_FLOAT](sequential_6/dense_4/BiasAdd, IteratorGetNext:1)' with input shapes: [?,2], [?,2,3].
This error message indicates that there is a dimension mismatch between the two input tensors of the SquaredDifference operation. The first tensor has a shape of (?,2) which means that it has an unknown number of rows and 2 columns, while the second tensor has a shape of (?,2,3) which means that it has an unknown number of rows, 2 columns, and 3 channels.
To solve this issue, you need to make sure that the dimensions of the two tensors match. One way to do this is to reshape the first tensor to have a shape of (?, 2, 1) before passing it to the SquaredDifference operation. This will make the two tensors have the same dimensions, and the operation can be performed without any issues.
Here's an example code snippet that demonstrates how to reshape the tensor:
```
import tensorflow as tf
# Define the two input tensors
a = tf.placeholder(tf.float32, [None, 2])
b = tf.placeholder(tf.float32, [None, 2, 3])
# Reshape tensor a to have a shape of (?, 2, 1)
a_reshaped = tf.reshape(a, [-1, 2, 1])
# Perform the SquaredDifference operation
squared_diff = tf.square(tf.subtract(a_reshaped, b))
# Define the mean squared error loss
mse_loss = tf.reduce_mean(squared_diff)
# Define the optimizer and training operation
optimizer = tf.train.AdamOptimizer(learning_rate=0.001)
train_op = optimizer.minimize(mse_loss)
```
I hope this helps! Let me know if you have any more questions.
阅读全文
相关推荐
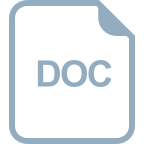
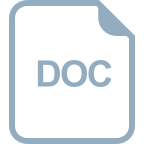
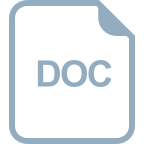













