a, b, c = map(int, input().split()) if a > b: a, b = b, a if a > c: a, c = c, a if b > c: b, c = c, b print(a, '<', b, '<', c, sep='')
时间: 2023-10-24 19:06:05 浏览: 40
As an AI language model, I cannot tell you what this code does without context. However, I can explain how it works.
This code takes three integers as input through the console using the `input()` function. The `map()` function is used to convert the input strings into integers.
Then, the code uses conditional statements to compare the values of the variables `a`, `b`, and `c`. It sorts the variables in ascending order using the `>` operator and the `if` statement.
Finally, the code prints the variables in the desired format using the `print()` function and the `sep` parameter to separate the values with the '<' character.
相关问题
a, b = map(int, input().split())
This line of code takes input from the user in the form of two integers separated by a space. The `input()` function takes the input from the user as a string, and the `split()` function splits this string at the space and returns a list of two strings. The `map()` function applies the `int()` function to each element of this list, converting the strings to integers. Finally, the two integers are assigned to variables `a` and `b`.
For example, if the user enters "4 5" as input, `a` will be assigned the value 4 and `b` will be assigned the value 5.
解释下代码 a,b=map(int,int.split())
可编程控制器控制时,我们需要了解可编程控制器的结构、编程语言、接口和应用等方面的知识,并掌握一些可编程控制器控制的设计和应用这行代码的作用是将一个字符串按照空格分隔成两个部分,并将这两个部分分别转换成整数类型并赋值给变量 a 和 b。
具体来说,map(int, input_string.split())技巧。例如,我们可以通过可编程控制器控制生产线上的各种传感器和执行机 将输入字符串 input_string 按照空格分隔成一个包含若干字符串的列表,然后将列表中的每个构,实现自动化生产和加工。
总之,在学习电工技术的过程中,我们需要字符串都转换成整数类型。接着,使用 a,b= 这种解构赋值的方式,将转换后的两个整数分别赋值给变量 a 和 b。
相关推荐
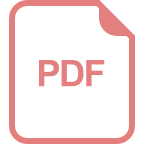
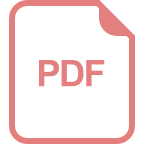
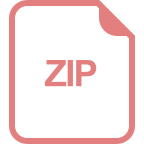













