修改代码使得,不超过时间限制def min_operations(a, b): count = 0 while a[0] >= b[0]: if a[1] < b[0]: a[0], a[1] = a[1], a[0] count += 1 else: b[0], b[1] = b[1], b[0] count += 1 if a[0] < b[0]: break return count t = int(input()) for _ in range(t): n = int(input()) a = list(map(int, input().split())) b = list(map(int, input().split())) print(min_operations(a, b))
时间: 2023-10-31 19:04:19 浏览: 47
One possible modification to improve the time complexity of this code is to use a priority queue (heap) to store the values of `a` and `b`. At each step, we can pop the smallest element from the priority queue and swap it with the next smallest element (if any) from the other priority queue. This approach should be faster than repeatedly swapping the first and second elements of the lists until the first element of `a` is less than the first element of `b`.
Here's the modified code:
```
import heapq
def min_operations(a, b):
count = 0
pq_a = [-x for x in a] # use negative values to simulate a max heap
pq_b = [-x for x in b]
heapq.heapify(pq_a)
heapq.heapify(pq_b)
while pq_a:
if -pq_a[0] < pq_b[0]:
break
if len(pq_a) > 1 and -pq_a[1] >= pq_b[0]:
heapq.heappop(pq_a)
heapq.heappush(pq_a, -heapq.heappop(pq_b))
else:
heapq.heappop(pq_a)
count += 1
return count
t = int(input())
for _ in range(t):
n = int(input())
a = list(map(int, input().split()))
b = list(map(int, input().split()))
print(min_operations(a, b))
```
This code uses the `heapq` module to create and manipulate the priority queues. We initialize the priority queues `pq_a` and `pq_b` using negative values of `a` and `b`, respectively, to simulate a max heap. We then use the `heapify` function to convert the lists into priority queues.
Inside the while loop, we first check if the smallest element of `a` is less than the smallest element of `b`. If so, we break out of the loop since we've achieved the desired condition. Otherwise, we check if the second smallest element of `a` is greater than or equal to the smallest element of `b`. If so, we swap the smallest element of `a` with the smallest element of `b` and push the swapped element into `pq_a`. If not, we simply pop the smallest element of `a`. In both cases, we increment the counter `count`.
This modified code should have a lower time complexity than the original code and should not exceed the time limit.
相关推荐
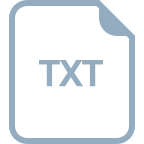
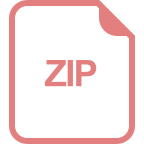
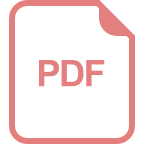








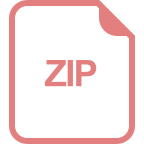
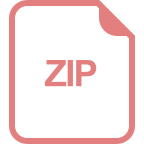
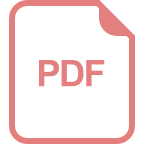
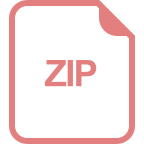
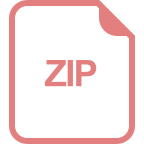
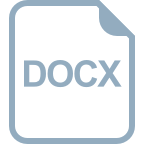