ValueError: Type mismatch (<class 'tuple'> vs. <class 'str'>) with values (() vs. coco_2017_train) for config key: DATASETS.TRAIN
时间: 2024-05-10 22:21:14 浏览: 317
This error occurs when there is a mismatch in the data type for the values assigned to the config key DATASETS.TRAIN. The expected data type for this key is a tuple, but the value assigned to it is a string.
To fix this error, you need to ensure that the value assigned to DATASETS.TRAIN is a tuple containing the required dataset information. For example, if you are using the COCO dataset, the correct value for DATASETS.TRAIN should be a tuple containing the dataset name and the dataset split, like so:
```
DATASETS.TRAIN = ('coco_2017_train', )
```
Make sure that you are using the correct data type for all the values assigned to the configuration keys in your code.
相关问题
ValueError: empty group <argparse._MutuallyExclusiveGroup object at 0x00000277889546D0>
这个错误提示通常出现在Python的argparse模块中,当你尝试处理命令行选项时,如果用户没有选择argparse中的互斥组(argparse._MutuallyExclusiveGroup)中的任何一个选项,就会抛出ValueError: empty group。argparse的互斥组是指一组选项必须至少选择其中一个,如果你的程序中设置了这样的约束,而用户没有提供满足条件的选择,就会报这个错。
例如,你可能有这样的代码结构:
```python
group = argparse.ArgumentParser().add_mutually_exclusive_group()
group.add_argument('--option1')
group.add_argument('--option2')
args = parser.parse_args()
```
在这种情况下,如果用户运行`python script.py`而没有指定`--option1`或`--option2`,就会触发这个错误。
解决办法是在解析命令行参数之前检查args.group是否为空,或者添加一个默认值或者帮助信息指导用户如何选择。
ValueError: Unknown type <class 'list'>
这个错误通常是因为你在使用一个需要特定类型参数的函数时,传递了错误的参数类型。可能的解决方案取决于你的具体代码和使用情况。
一些常见的解决方案包括检查你的代码,确保你正在传递正确的参数类型,如果需要,可以尝试将参数转换为正确的类型。另外,你也可以查看函数的文档或源代码,确定它所需的参数类型,并确保你的代码与之匹配。如果这些解决方案都无法解决问题,你可以提供更多的上下文或代码,以便更好地理解问题并提供更精确的解决方案。
阅读全文
相关推荐
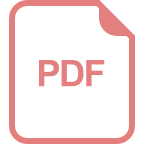
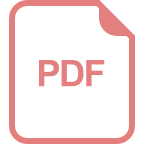
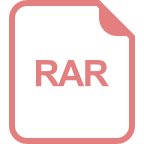













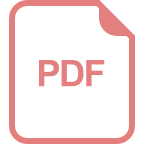