用c plus plus 14写一个函数快速清空priority_queue变量
时间: 2023-06-03 08:07:41 浏览: 117
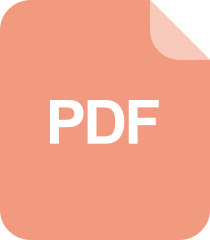
优先队列(priority_queue)的C语言实现代码
好的,以下是可以回答的代码段:
```c++
template<class T> void clear(priority_queue<T>& q) {
priority_queue<T> empty;
swap(q, empty);
}
```
请注意,以上是一份 c++14 代码,可以快速清空 priority_queue 变量。如果你有任何其他问题,欢迎继续提问。
阅读全文
相关推荐




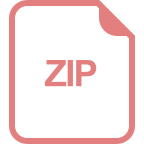
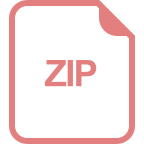







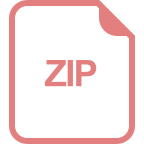
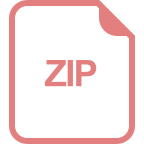