使用weighted Voronoi stippling 和opencv库生成tsp文件代码
时间: 2024-05-12 15:13:22 浏览: 11
下面是使用weighted Voronoi stippling 和 OpenCV 库生成 TSP 文件的 Python 代码:
```python
import cv2
import numpy as np
import math
# 定义点类
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
# 计算两点之间的欧几里得距离
def distance(p1, p2):
return math.sqrt((p1.x - p2.x) ** 2 + (p1.y - p2.y) ** 2)
# 生成 weighted Voronoi 图
def generate_voronoi(img, points):
# 创建一个空白图像,用于绘制 Voronoi 图
voronoi_img = np.zeros(img.shape, dtype=np.uint8)
# 创建一个 Subdiv2D 对象,并插入点
subdiv = cv2.Subdiv2D((0, 0, img.shape[1], img.shape[0]))
for p in points:
subdiv.insert((p.x, p.y))
# 获取 Voronoi 图中的所有边界和顶点
(edges, vertices) = subdiv.getVoronoiFacetList([])
# 绘制 Voronoi 图
for i in range(len(edges)):
# 获取 Voronoi 图中的两个顶点
pt1 = (int(vertices[edges[i][0]][0]), int(vertices[edges[i][0]][1]))
pt2 = (int(vertices[edges[i][1]][0]), int(vertices[edges[i][1]][1]))
# 绘制 Voronoi 边界
cv2.line(voronoi_img, pt1, pt2, (255, 255, 255), 1)
return voronoi_img
# 生成 TSP 文件
def generate_tsp_file(points, filename):
tsp_file = open(filename, "w")
# 写入 TSP 文件头
tsp_file.write("NAME: weighted_voronoi\n")
tsp_file.write("TYPE: TSP\n")
tsp_file.write("COMMENT: Weighted Voronoi diagram\n")
tsp_file.write("DIMENSION: " + str(len(points)) + "\n")
tsp_file.write("EDGE_WEIGHT_TYPE: EUC_2D\n")
tsp_file.write("NODE_COORD_SECTION\n")
# 写入节点坐标
for i in range(len(points)):
tsp_file.write(str(i + 1) + " " + str(points[i].x) + " " + str(points[i].y) + "\n")
# 写入 TSP 文件尾
tsp_file.write("EOF\n")
tsp_file.close()
# 读取输入图像
img = cv2.imread("input.png", cv2.IMREAD_GRAYSCALE)
# 对输入图像进行二值化处理
ret, img = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)
# 使用 weighted Voronoi stippling 生成点
points = []
for y in range(img.shape[0]):
for x in range(img.shape[1]):
if img[y, x] == 255:
weight = np.sum(img[max(y - 3, 0):min(y + 4, img.shape[0]), max(x - 3, 0):min(x + 4, img.shape[1])] == 255)
if weight > 0:
points.append(Point(x, y))
# 生成 weighted Voronoi 图
voronoi_img = generate_voronoi(img, points)
# 保存 weighted Voronoi 图
cv2.imwrite("voronoi.png", voronoi_img)
# 生成 TSP 文件
generate_tsp_file(points, "weighted_voronoi.tsp")
```
上述代码的输入图像应该命名为 "input.png" ,且它应该是一个单通道灰度图像。该代码使用 `cv2.imread` 函数读取输入图像,并使用 `cv2.threshold` 函数将其转换为二值图像。然后,使用 weighted Voronoi stippling 生成点,并使用 `generate_voronoi` 函数生成 weighted Voronoi 图。最后,使用 `generate_tsp_file` 函数生成 TSP 文件。TSP 文件将被保存在当前目录下,命名为 "weighted_voronoi.tsp"。
相关推荐
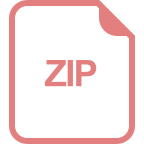
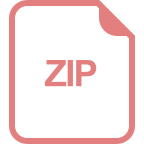














