用c++合并两个无序链表
时间: 2023-05-31 13:04:45 浏览: 105
以下是使用C语言合并两个无序链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node* createList(int arr[], int n) {
Node *head = NULL, *tail = NULL;
for (int i = 0; i < n; i++) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
if (tail == NULL) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
return head;
}
void printList(Node *head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
Node* mergeLists(Node *head1, Node *head2) {
if (head1 == NULL) {
return head2;
}
if (head2 == NULL) {
return head1;
}
Node *ptr1 = head1, *ptr2 = head2;
Node *head = NULL, *tail = NULL;
while (ptr1 != NULL && ptr2 != NULL) {
Node *newNode = (Node*) malloc(sizeof(Node));
if (ptr1->data <= ptr2->data) {
newNode->data = ptr1->data;
ptr1 = ptr1->next;
} else {
newNode->data = ptr2->data;
ptr2 = ptr2->next;
}
newNode->next = NULL;
if (tail == NULL) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
if (ptr1 != NULL) {
tail->next = ptr1;
}
if (ptr2 != NULL) {
tail->next = ptr2;
}
return head;
}
int main() {
int arr1[] = {3, 1, 4, 5, 2};
int arr2[] = {9, 6, 7, 8};
Node *head1 = createList(arr1, 5);
Node *head2 = createList(arr2, 4);
printf("List 1: ");
printList(head1);
printf("List 2: ");
printList(head2);
Node *head = mergeLists(head1, head2);
printf("Merged List: ");
printList(head);
return 0;
}
```
在上面的代码中,我们定义了一个`Node`结构体来表示链表的节点,其中包含一个整数数据和一个指向下一个节点的指针。我们还定义了`createList`函数来创建链表,`printList`函数来打印链表,以及`mergeLists`函数来合并两个链表。
`mergeLists`函数使用两个指针`ptr1`和`ptr2`分别遍历两个链表,并将它们的节点逐个比较,将较小的节点添加到新链表中。最后,将任何剩余的节点添加到新链表的末尾,并返回新链表的头指针。
在`main`函数中,我们创建了两个无序链表,打印它们,然后将它们合并并打印结果链表。运行程序将输出以下内容:
```
List 1: 3 1 4 5 2
List 2: 9 6 7 8
Merged List: 1 2 3 4 5 6 7 8 9
```
相关推荐
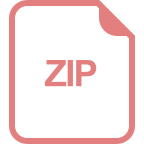
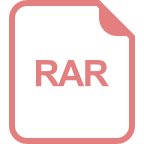














