用Java编写GUI界面,模拟抢红包游戏,在界面输入红包大小和抢红包人姓名(用逗号隔开),输出系统当前时间以及每个人抢的红包大小,要求每个人抢的红包最少不能少于0.01元,精确到小数点后两位
时间: 2024-04-05 10:29:20 浏览: 13
好的,这是一个很有趣的项目!首先,我们需要创建一个 Java GUI 窗口来接收用户输入和显示输出结果。你可以使用Swing或JavaFX等库来创建GUI界面。下面是一个简单的Swing实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
public class RedPacketGame extends JFrame implements ActionListener {
private JLabel amountLabel, nameLabel, timeLabel;
private JTextField amountText, nameText;
private JButton addButton, startButton;
private JTextArea resultArea;
private ArrayList<String> names;
private ArrayList<Double> amounts;
public RedPacketGame() {
super("模拟抢红包游戏");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 500);
setResizable(false);
// 创建GUI组件
amountLabel = new JLabel("红包大小:");
nameLabel = new JLabel("抢红包人:");
timeLabel = new JLabel();
amountText = new JTextField(10);
nameText = new JTextField(30);
addButton = new JButton("添加");
startButton = new JButton("开始");
resultArea = new JTextArea(20, 40);
names = new ArrayList<>();
amounts = new ArrayList<>();
// 添加GUI组件
JPanel inputPanel = new JPanel();
inputPanel.add(amountLabel);
inputPanel.add(amountText);
inputPanel.add(nameLabel);
inputPanel.add(nameText);
inputPanel.add(addButton);
inputPanel.add(startButton);
inputPanel.add(timeLabel);
JScrollPane scrollPane = new JScrollPane(resultArea);
add(inputPanel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
// 添加事件监听器
addButton.addActionListener(this);
startButton.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
addRedPacket();
} else if (e.getSource() == startButton) {
startGame();
}
}
private void addRedPacket() {
String inputAmount = amountText.getText().trim();
String inputNames = nameText.getText().trim();
if (inputAmount.equals("") || inputNames.equals("")) {
JOptionPane.showMessageDialog(this, "请输入红包大小和抢红包人姓名");
return;
}
double amount = 0;
try {
amount = Double.parseDouble(inputAmount);
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "请输入正确的红包大小");
return;
}
if (amount <= 0) {
JOptionPane.showMessageDialog(this, "红包大小必须大于0");
return;
}
String[] namesArray = inputNames.split(",");
for (String name : namesArray) {
name = name.trim();
if (name.equals("")) {
JOptionPane.showMessageDialog(this, "请输入正确的抢红包人姓名");
return;
}
names.add(name);
amounts.add(0.0);
}
amountText.setText("");
nameText.setText("");
JOptionPane.showMessageDialog(this, "添加成功");
}
private void startGame() {
if (names.isEmpty()) {
JOptionPane.showMessageDialog(this, "请添加抢红包人");
return;
}
double leftAmount = Double.parseDouble(amountText.getText().trim());
if (leftAmount <= 0) {
JOptionPane.showMessageDialog(this, "红包大小必须大于0");
return;
}
resultArea.setText("");
while (leftAmount > 0) {
for (int i = 0; i < names.size(); i++) {
if (leftAmount <= 0) {
break;
}
double amount = Math.random() * leftAmount;
if (amount < 0.01) {
amount = 0.01;
}
amounts.set(i, amounts.get(i) + amount);
leftAmount -= amount;
resultArea.append(names.get(i) + " 抢到了 " + String.format("%.2f", amount) + " 元\n");
try {
Thread.sleep(500); // 等待0.5秒
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
}
resultArea.append("红包已被抢完\n");
for (int i = 0; i < names.size(); i++) {
resultArea.append(names.get(i) + " 抢到了 " + String.format("%.2f", amounts.get(i)) + " 元\n");
}
// 显示系统当前时间
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
timeLabel.setText("当前时间:" + sdf.format(new Date()));
addButton.setEnabled(false);
startButton.setEnabled(false);
}
public static void main(String[] args) {
RedPacketGame game = new RedPacketGame();
game.setVisible(true);
}
}
```
这个程序包含了一个 JFrame 窗口,用户可以在上面输入红包大小和抢红包人姓名,然后点击“添加”按钮来添加抢红包人。在添加完所有抢红包人后,用户可以点击“开始”按钮来开始模拟抢红包游戏。程序会随机生成每个人抢到的红包金额,并在界面上显示出来。当红包被抢完后,程序会显示每个人最终抢到的金额,并显示当前系统时间。
在上面的代码中,我们使用了一个 ArrayList 来存储抢红包人的姓名和抢到的金额。为了保证每个人抢到的金额最少为0.01元,我们在生成随机金额时,如果生成的金额小于0.01元,则将其设置为0.01元。此外,为了让程序的运行看起来更加真实,我们在每次生成随机金额后,让程序等待0.5秒再继续运行。
希望这个程序能够满足你的需求!如果你有其他问题或者需要进一步的帮助,请随时联系我。
相关推荐
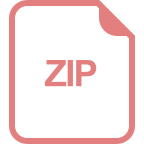
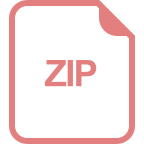
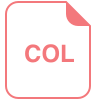

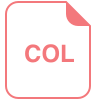
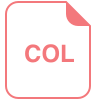











